This C program calculates the simple interest based on the principal amount, interest rate, and time period. It applies the formula: simple_interest = (principal * rate * time) / 100 and provides the resulting value.
In finance, interest refers to the cost of borrowing money or the return on investment when lending money. Simple interest is a straightforward method used to calculate interest on a loan or investment, based on the principal amount, the interest rate, and the time period involved.
Program Statement
Write a C program to calculate the simple interest based on the principle amount, rate of interest, and time period provided by the user. Display the calculated simple interest as the output.
The program should perform the following steps:
- Prompt the user to enter the principle amount.
- Read and store the principle amount.
- Prompt the user to enter the rate of interest.
- Read and store the rate of interest.
- Prompt the user to enter the time period in years.
- Read and store the time period.
- Calculate the simple interest using the formula: (principle * rate * time) / 100.
- Display the calculated simple interest on the screen.
C Program to Find Simple Interest
#include <stdio.h> int main() { float principal, rate, time, interest; // Input the principal amount, rate of interest, and time printf("Enter the principal amount: "); scanf("%f", &principal); printf("Enter the rate of interest (in percentage): "); scanf("%f", &rate); printf("Enter the time period (in years): "); scanf("%f", &time); // Calculate the simple interest interest = (principal * rate * time) / 100; // Print the simple interest printf("Simple Interest = %.2f\n", interest); return 0; }
How it works?
- The program starts by including the necessary header file,
stdio.h
, which provides input and output functions. - Inside the
main()
function, the program declares the necessary variables:principle
,rate
,time
, andinterest
, all of which are of typefloat
. - The program prompts the user to enter the principle amount using
printf()
. - The
scanf()
function is used to read and store the principle amount entered by the user in the variableprinciple
. - The program then prompts the user to enter the rate of interest.
- The
scanf()
function is used again to read and store the rate of interest entered by the user in the variablerate
. - Next, the program prompts the user to enter the time period in years.
- The
scanf()
function is used once more to read and store the time period entered by the user in the variabletime
. - The program calculates the simple interest using the formula
(principle * rate * time) / 100
and stores the result in the variableinterest
. - Finally, the program uses
printf()
to display the calculated simple interest on the screen, with the value being rounded to two decimal places using the%.2f
format specifier. - The program ends with the
return 0;
statement, indicating successful execution.
By following these steps, the program takes user inputs for the principle amount, rate of interest, and time period, calculates the simple interest using the provided formula, and displays the result.
Input/Output
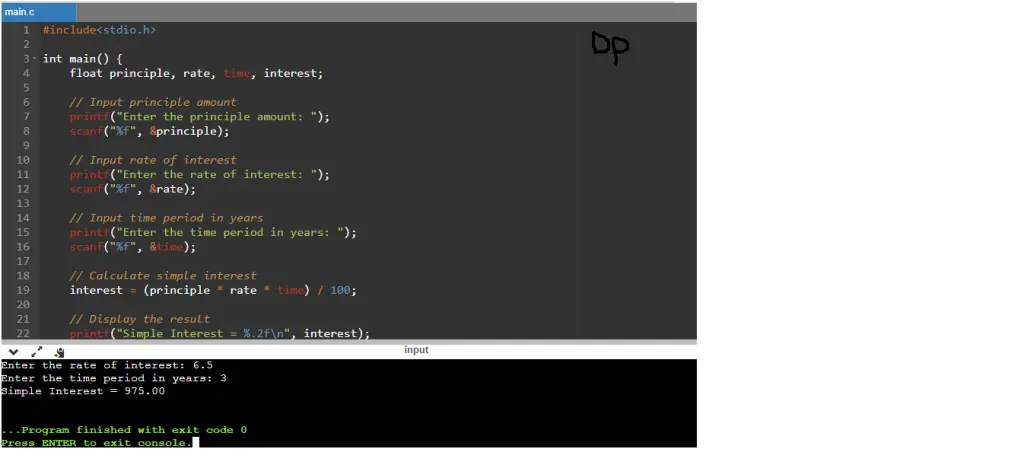