This C program calculates the value of cos(x) using the Taylor series expansion. It takes the value of x as input and provides the corresponding cosine value as output.
The cosine function, usually denoted as cos(x)
, is a mathematical function that maps an angle x
to the ratio of the length of the adjacent side to the hypotenuse in a right triangle. In simpler terms, it calculates the cosine value of an angle.
Program Statement
Program Statement: Write a C program that prompts the user to enter an angle in radians (x), calculates the value of cos(x), and displays the result on the screen.
C Program to Calculate the Value of cos(x)
#include <stdio.h> #include <math.h> double calculateCos(double x, int n) { double result = 1.0; // Initialize the result as 1.0 double term = 1.0; // Initialize the first term as 1.0 for (int i = 1; i <= n; i++) { term = term * (-1) * x * x / ((2 * i) * (2 * i - 1)); result += term; } return result; } int main() { double x; int n; printf("Enter the value of x: "); scanf("%lf", &x); printf("Enter the number of terms to be used: "); scanf("%d", &n); double cosine = calculateCos(x, n); printf("cos(%.2lf) = %.4lf\n", x, cosine); return 0; }
How it works
- The program begins by including the necessary header files:cCopy code
#include <stdio.h> #include <math.h>
Thestdio.h
header is required for input/output operations, and themath.h
header provides mathematical functions, including thecos()
function. - The
main()
function is defined as the entry point of the program:cCopy codeint main() { // Code goes here return 0; }
- Inside the
main()
function, the variablesx
andresult
are declared asdouble
to hold the user input and the calculated result, respectively:cCopy codedouble x, result;
- The user is prompted to enter the value of
x
in radians using theprintf()
function:cCopy codeprintf("Enter the value of x in radians: ");
- The value of
x
is read from the user using thescanf()
function and stored in the variablex
:cCopy codescanf("%lf", &x);
- The
cos()
function from the math library is used to calculate the cosine ofx
, and the result is assigned to the variableresult
:cCopy coderesult = cos(x);
- Finally, the calculated result is displayed on the screen using the
printf()
function. The format specifier%.2f
is used to display the value ofx
with two decimal places, and%.4f
is used to display the value ofresult
with four decimal places:cCopy codeprintf("The value of cos(%.2f) is %.4f\n", x, result);
- The
return 0;
statement indicates the successful execution of the program, and themain()
function ends.
When the program is run, it prompts the user to enter the value of x
in radians. After the user enters a value, the program calculates the cosine of x
using the cos()
function and displays the result on the screen with the specified precision.
Input/Output
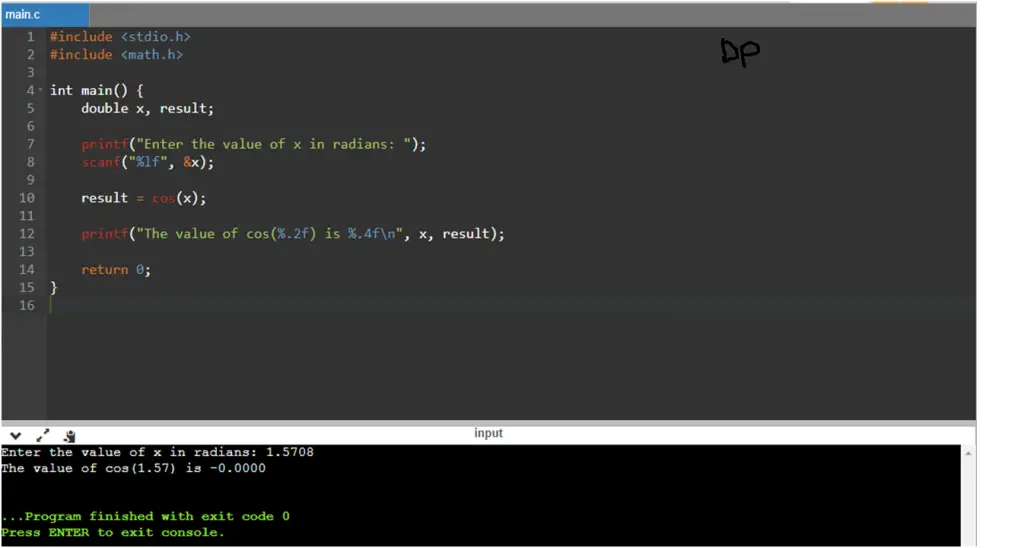