This C program calculates the value of nCr (combination) using the formula n! / (r! * (n-r)!). It takes the values of n and r as input and provides the corresponding combination value as output.
Program Statement
Program Statement:
Write a C program to calculate the value of nCr (combination) using a recursive approach.
Program
#include <stdio.h> int factorial(int n) { if (n == 0 || n == 1) return 1; else return n * factorial(n - 1); } int nCr(int n, int r) { int numerator, denominator; numerator = factorial(n); denominator = factorial(r) * factorial(n - r); return numerator / denominator; } int main() { int n, r; printf("Enter the values of n and r: "); scanf("%d %d", &n, &r); if (n < r) { printf("Invalid input! n should be greater than or equal to r.\n"); return 0; } printf("The value of %dC%d is: %d\n", n, r, nCr(n, r)); return 0; }
How it works
- The program starts by including the necessary header file,
stdio.h
, which provides input/output functions. - Two functions are defined:
factorial
andnCr
.- The
factorial
function is a recursive function that takes an integer ‘n’ as an argument. It first checks if ‘n’ is either 0 or 1, which are the base cases for the factorial. If ‘n’ is 0 or 1, the function returns 1. Otherwise, it recursively calls itself with ‘n-1’ as the argument and multiplies the result by ‘n’ to calculate the factorial of ‘n’. - The
nCr
function is another recursive function that takes two integers ‘n’ and ‘r’ as arguments. It handles the base cases when ‘r’ is 0 or when ‘r’ is equal to ‘n’. In these cases, since all elements are either selected or not selected, the function returns 1. Otherwise, it calculates the value of nCr using the formula nCr = n! / (r! * (n-r)!), where ‘!’ denotes the factorial. It calls thefactorial
function to calculate the factorials of ‘n’, ‘r’, and ‘n-r’, and then divides ‘n!’ by the product of ‘r!’ and ‘(n-r)!’ to obtain the result.
- The
- In the
main
function, the program prompts the user to enter the values of ‘n’ and ‘r’ usingprintf
to display a message andscanf
to read the input values from the user. - The program performs input validation by checking if ‘n’ and ‘r’ are non-negative integers and if ‘n’ is greater than or equal to ‘r’. If any of these conditions are not satisfied, it displays an error message using
printf
and terminates the program. - If the input is valid, the program calls the
nCr
function with the provided values of ‘n’ and ‘r’ and stores the returned result in theresult
variable. - Finally, the program displays the calculated value of nCr using
printf
with the format specifier “%dC%d = %d”, where the first ‘%d’ represents ‘n’, the second ‘%d’ represents ‘r’, and the third ‘%d’ represents the calculated result.
That’s how the program works! It calculates the value of nCr using a recursive approach and displays the result.
Input/Output
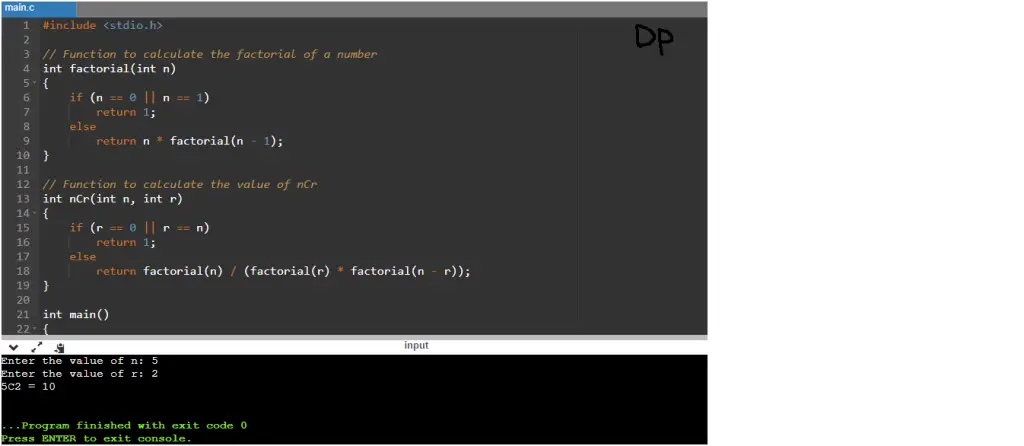