This C program calculates the volume and surface area of a cuboid given its length, width, and height. It utilizes the formulas for volume and surface area and provides the results based on the provided dimensions.
A cuboid is a three-dimensional geometric shape that has six rectangular faces. It is also known as a rectangular prism. The volume and surface area are important properties of a cuboid, and they can be calculated using simple formulas.
Program Statement
Write a C program to find the volume and surface area of a cuboid
Program
#include<stdio.h> int main() { float length, width, height; float volume, surface_area; printf("Enter the length of the cuboid: "); scanf("%f", &length); printf("Enter the width of the cuboid: "); scanf("%f", &width); printf("Enter the height of the cuboid: "); scanf("%f", &height); // Calculate the volume of the cuboid volume = length * width * height; // Calculate the surface area of the cuboid surface_area = 2 * (length * width + length * height + width * height); printf("Volume of the cuboid: %.2f\n", volume); printf("Surface area of the cuboid: %.2f\n", surface_area); return 0; }
How it works
Here’s a step-by-step explanation of how the program works:
- The program starts by declaring the necessary variables:
length
,width
,height
,volume
, andsurfaceArea
, all of which are of typefloat
. - The user is prompted to enter the length of the cuboid using
printf
. - The program uses
scanf
to read and store the length entered by the user in the variablelength
. - Similarly, the user is prompted to enter the width of the cuboid.
- The program uses
scanf
to read and store the width entered by the user in the variablewidth
. - The user is prompted to enter the height of the cuboid.
- The program uses
scanf
to read and store the height entered by the user in the variableheight
. - Using the values of
length
,width
, andheight
, the program calculates the volume of the cuboid using the formula:volume = length * width * height
. - Similarly, the program calculates the surface area of the cuboid using the formula:
surfaceArea = 2 * (length * width + length * height + width * height)
. - The program then uses
printf
to display the calculated volume and surface area to the user with appropriate labels. - Finally, the program ends, and the execution is complete.
By following these steps, the program takes the user’s input for the dimensions of the cuboid, performs the necessary calculations, and provides the volume and surface area of the cuboid as output
Input/Output
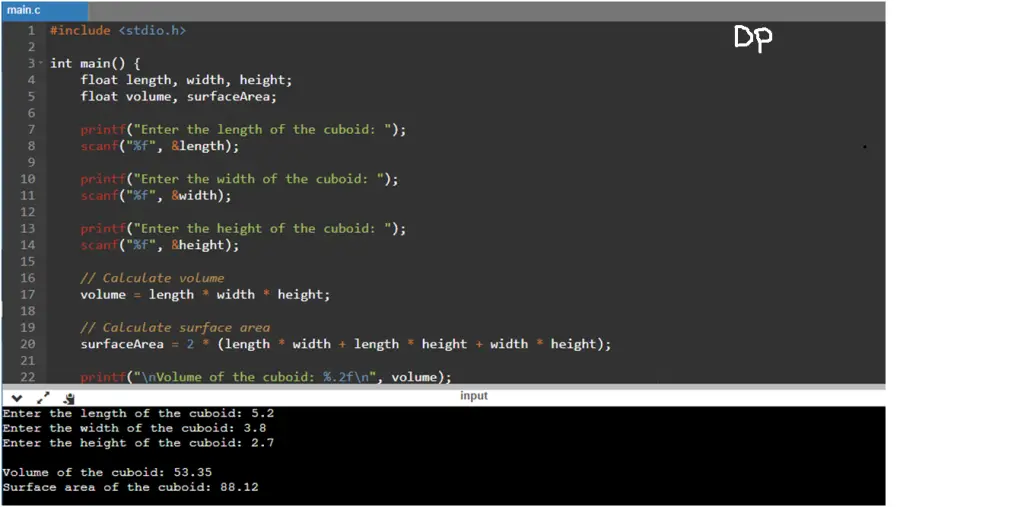