This C program calculates the compound interest for a given principal amount, interest rate, and time period. It utilizes the formula for compound interest and provides the resulting amount at the end of the given time period.
Compound interest is a concept in finance that involves the growth of an initial investment over time. Unlike simple interest, which is calculated only on the principal amount, compound interest takes into account both the principal and the accumulated interest from previous periods.
C Program to Find Compound Interest
Program Statement: Write a C program to calculate compound interest. The program should prompt the user to enter the principal amount, interest rate (in decimal form), and time period (in years). It should then calculate and display the compound interest based on the given inputs using the formula:
A = P * (1 + r/n)^(n*t)
where:
- A is the total amount including principal and interest
- P is the principal amount (initial investment)
- r is the annual interest rate (expressed as a decimal)
- n is the number of times interest is compounded per year
- t is the number of years
The program should display the calculated compound interest with two decimal places.
Program
#include <stdio.h> #include <math.h> double calculateCompoundInterest(double principal, double rate, int time) { double amount = principal * pow((1 + rate / 100), time); double compoundInterest = amount - principal; return compoundInterest; } int main() { double principal, rate; int time; printf("Enter principal amount: "); scanf("%lf", &principal); printf("Enter rate of interest: "); scanf("%lf", &rate); printf("Enter time (in years): "); scanf("%d", &time); double compoundInterest = calculateCompoundInterest(principal, rate, time); printf("Compound Interest = %.2lf\n", compoundInterest); return 0; }
How it works
- The program starts by including the necessary header files:
stdio.h
for input/output functions andmath.h
for mathematical functions. - The
main()
function is defined as the entry point of the program. - Inside the
main()
function, variables are declared to store the principal amount, interest rate, time period, and compound interest. - The program prompts the user to enter the principal amount using
printf()
. - The
scanf()
function is used to read the principal amount entered by the user and store it in theprincipal
variable. - Similarly, the program prompts the user to enter the interest rate and time period, and the entered values are stored in the
rate
andtime
variables, respectively. - The compound interest is calculated using the formula
compound_interest = principal * pow((1 + rate), time)
.- The
pow()
function from themath.h
library is used to calculate the power of(1 + rate)
raised to thetime
exponent. This calculates the effect of compounding on the principal amount over the given time period.
- The
- The calculated compound interest is displayed to the user using
printf()
.- The format specifier
%.2lf
is used to display the compound interest with two decimal places.
- The format specifier
- The program ends by returning 0 from the
main()
function, indicating successful execution.
That’s how the program works! It prompts the user for input, calculates the compound interest based on the provided values, and then displays the result.
Input/Output
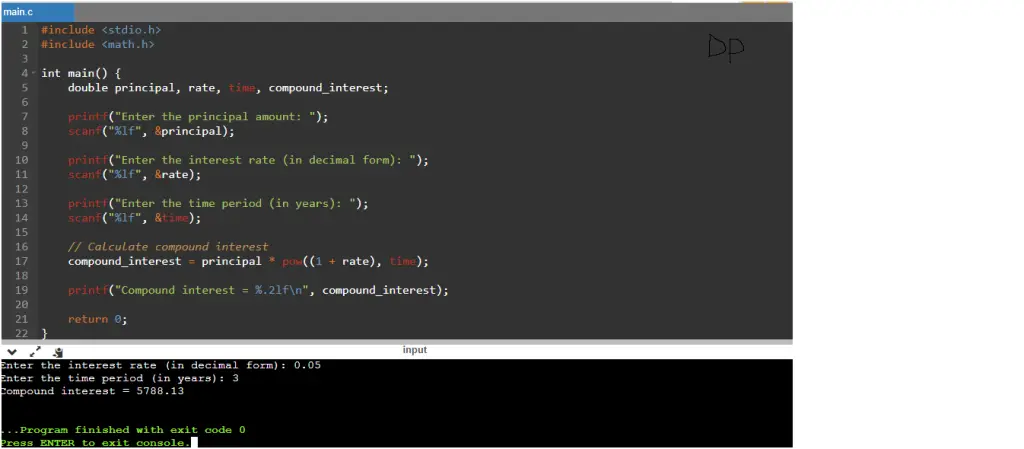