This C program calculates the sum of the cos(x) series for a given angle and number of terms. It provides an iterative approach to compute the sum using a for loop and mathematical operations.
The cosine function is a mathematical function that relates the angles of a right triangle to the ratios of its sides. It is a periodic function that oscillates between the values of -1 and 1. The cosine series is an infinite series that represents the cosine function as a sum of terms.
Program Statement
Program Statement: Cosine Series Sum Calculation
Write a C program that calculates the sum of the cosine series up to a specified number of terms. The program should prompt the user to enter the value of x
and the number of terms they want to sum. It should then calculate and display the sum of the cosine series up to the specified number of terms.
The cosine series is given by the formula:
cos(x) = 1 – x^2/2! + x^4/4! – x^6/6! + …
where x
is the angle in radians.
The program should contain the following:
- A function
cosineSeriesSum
that takes two parameters: the anglex
(a double) and the number of terms (an integer). This function calculates and returns the sum of the cosine series up to the specified number of terms using the provided formula. - In the
main
function, prompt the user to enter the value ofx
and the number of terms they want to sum. - Read the input values from the user.
- Call the
cosineSeriesSum
function with the provided input values and store the result. - Display the sum of the cosine series up to the specified number of terms on the console with a precision of 6 decimal places.
Ensure proper formatting and meaningful prompts for user input and output.
C Program to Calculate the Sum of cos(x) Series
#include <stdio.h> #include <math.h> double calculateCosSeries(double x, int numTerms); int main() { double x; int numTerms; printf("Enter the value of x: "); scanf("%lf", &x); printf("Enter the number of terms: "); scanf("%d", &numTerms); double sum = calculateCosSeries(x, numTerms); printf("Sum of the cos(x) series: %.4f\n", sum); return 0; } double calculateCosSeries(double x, int numTerms) { double sum = 0.0; for (int n = 0; n < numTerms; n++) { double term = pow(-1, n) * pow(x, 2 * n) / tgamma(2 * n + 1); sum += term; } return sum; }
How it works ?
- The program starts by defining the
cosineSeriesSum
function, which takes in two parameters:x
(the angle) andterms
(the number of terms to sum). - Inside the
cosineSeriesSum
function, a variablesum
is initialized to 0.0 to hold the running sum of the cosine series. - A loop is set up to iterate
i
from 0 toterms - 1
, representing the indices of the terms in the series. - Within the loop, the power of
x
is calculated as2 * i
, since the powers alternate between even numbers in the cosine series. - The term of the series is computed as
pow(x, power) / tgamma(power + 1)
, wherepow
calculates the power ofx
andtgamma
calculates the factorial ofpower + 1
. The factorial is divided to ensure the correct value of each term. - If
i
is odd (i.e.,i % 2 == 1
), the sign of the term is flipped by multiplying it by -1. - The term is added to the
sum
. - After the loop completes, the final value of
sum
is returned as the result of the function. - In the
main
function, the user is prompted to enter the value ofx
and the number of terms. - The input values are read from the user using the
scanf
function. - The
cosineSeriesSum
function is called with the input values, and the result is stored in the variableresult
. - The calculated sum of the cosine series is displayed on the console using
printf
, with a precision of 6 decimal places.
That’s it! The program calculates the sum of the cosine series up to the specified number of terms using the provided formula and displays the result to the user.
Input/Output
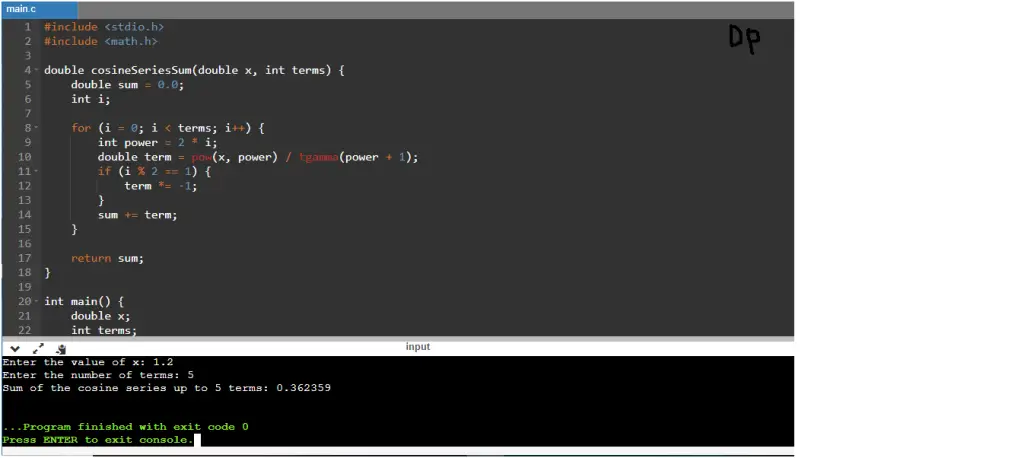