This C program finds the first N Fibonacci numbers and displays them.
Problem statement
Write a C program to find the first N Fibonacci numbers.
C Program to Find First N Fibonacci Numbers
#include <stdio.h> void fibonacci(int n) { int i, t1 = 0, t2 = 1, nextTerm; printf("The first %d Fibonacci numbers are: ", n); for (i = 1; i <= n; ++i) { printf("%d, ", t1); nextTerm = t1 + t2; t1 = t2; t2 = nextTerm; } } int main() { int n; printf("Enter the value of N: "); scanf("%d", &n); fibonacci(n); return 0; }
How it works?
- The program defines a function
fibonacci
that takes an integern
as input. - Inside the
fibonacci
function, two variablest1
andt2
are initialized to the first two Fibonacci numbers, 0 and 1. - The program then enters a loop that runs
n
times. In each iteration, it prints the current Fibonacci number (t1
), calculates the next Fibonacci number by addingt1
andt2
, and updates the values oft1
andt2
accordingly. - The loop continues until the desired number of Fibonacci numbers are printed.
- In the
main
function, the user is prompted to enter the value ofN
. - The input value is read using
scanf
and stored in the variablen
. - Finally, the
fibonacci
function is called with the value ofn
as an argument.
Input/Output
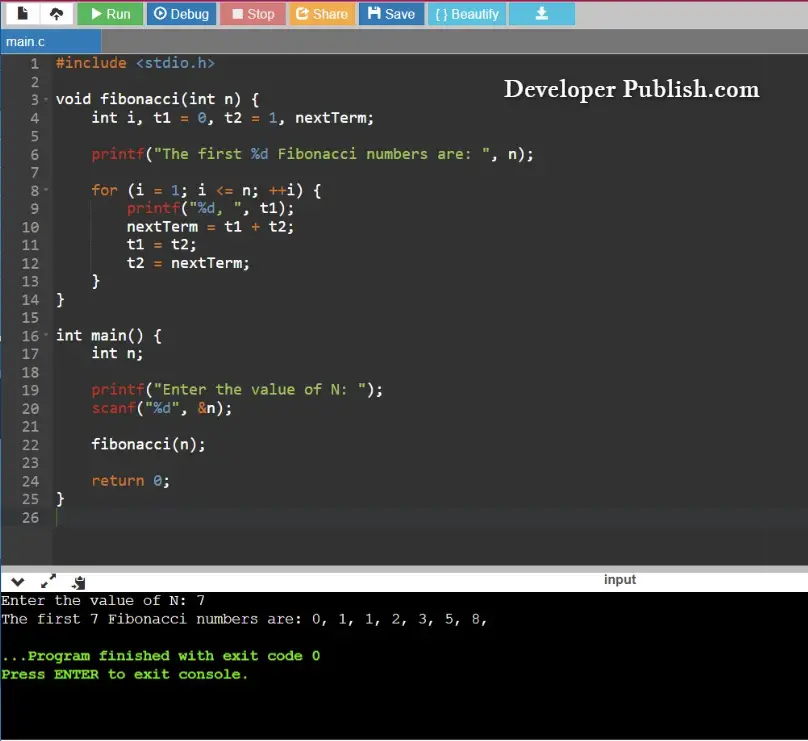