This C program implements the Fizzbuzz problem, a classic programming exercise. It prints numbers from 1 to a given limit, replacing multiples of 3 with “Fizz”, multiples of 5 with “Buzz”,
and multiples of both 3 and 5 with “FizzBuzz”.
The rules of FizzBuzz are straightforward:
- Print “Fizz” if the number is divisible by 3.
- Print “Buzz” if the number is divisible by 5.
- Print “FizzBuzz” if the number is divisible by both 3 and 5.
- If none of the above conditions are met, simply print the number itself.
The objective of the FizzBuzz problem is to write a program that generates this sequence of numbers and prints the appropriate word or number for each iteration.
Problem Statement
The FizzBuzz problem is a common programming task that asks you to write a program that prints the numbers from 1 to 100. However, there are a few exceptions:
- For numbers divisible by 3, instead of the number, you should print “Fizz”.
- For numbers divisible by 5, instead of the number, you should print “Buzz”.
- For numbers divisible by both 3 and 5, instead of the number, you should print “FizzBuzz”.
The task is to write a program that implements this behavior and prints the numbers from 1 to 100 according to the rules described above.
Fizzbuzz Program in C Programming
#include <stdio.h> int main() { int i; for (i = 1; i <= 100; i++) { if (i % 3 == 0 && i % 5 == 0) { printf("FizzBuzz\n"); } else if (i % 3 == 0) { printf("Fizz\n"); } else if (i % 5 == 0) { printf("Buzz\n"); } else { printf("%d\n", i); } } return 0; }
How it works
The program starts a loop that iterates through numbers from 1 to 100 (or any given limit). It uses afor
loop or a similar looping construct to go through each number in the sequence.- For each number, the program checks three conditions using the modulus operator (
%
):- If the number is divisible by 3 (i.e., the remainder of the division by 3 is 0), it prints “Fizz”.
- If the number is divisible by 5, it prints “Buzz”.
- If the number is divisible by both 3 and 5, it prints “FizzBuzz”.
- If none of the above conditions are met, it simply prints the number itself.
- The program continues the loop, repeating the above steps for each number in the sequence until it reaches the given limit (in this case, 100).
By applying these rules to each number in the sequence, the program generates the FizzBuzz sequence, where multiples of 3 are replaced with “Fizz”, multiples of 5 are replaced with “Buzz”, and multiples of both 3 and 5 are replaced with “FizzBuzz”.
Input/Output
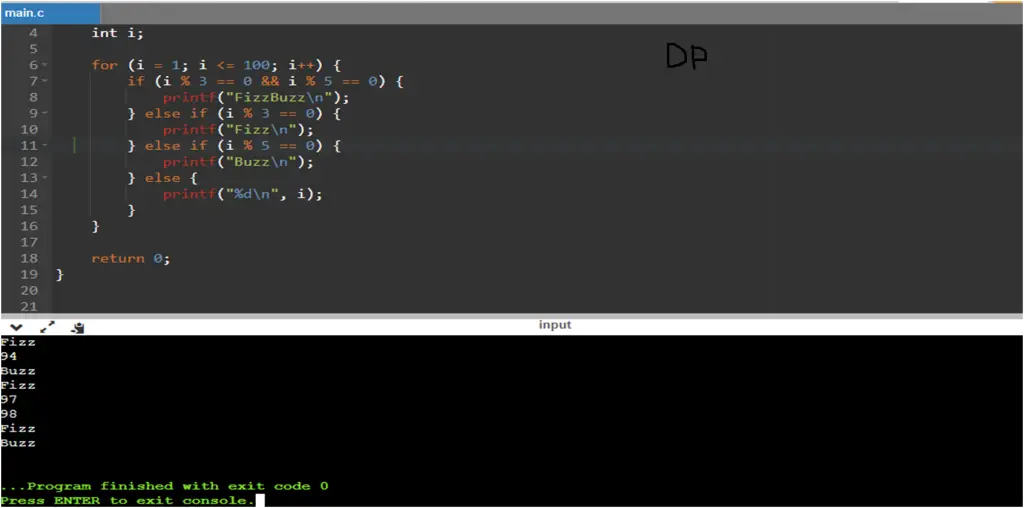