Boxing and unboxing are fundamental concepts in C# related to the manipulation of value types and reference types.
Problem Statement
You are tasked with implementing a simple application that demonstrates the concepts of boxing and unboxing in C#.
C# Program to Demonstrate Boxing Operations
using System; class Program { static void Main() { // Boxing: Converting an int to an object int intValue = 42; object boxedValue = intValue; // Unboxing: Converting an object back to int int unboxedValue = (int)boxedValue; Console.WriteLine("Original int value: " + intValue); Console.WriteLine("Boxed object value: " + boxedValue); Console.WriteLine("Unboxed int value: " + unboxedValue); // Boxing with other value types double doubleValue = 3.14159265359; object boxedDouble = doubleValue; // Unboxing the double double unboxedDouble = (double)boxedDouble; Console.WriteLine("Original double value: " + doubleValue); Console.WriteLine("Boxed double value: " + boxedDouble); Console.WriteLine("Unboxed double value: " + unboxedDouble); // Demonstrating boxing with value types bool boolValue = true; object boxedBool = boolValue; // Unboxing the boolean bool unboxedBool = (bool)boxedBool; Console.WriteLine("Original bool value: " + boolValue); Console.WriteLine("Boxed bool value: " + boxedBool); Console.WriteLine("Unboxed bool value: " + unboxedBool); } }
How it Works
Boxing:
- Create a Value Type: In boxing, you start with a value type variable. For example, let’s consider an
int
variable namedintValue
, which holds the value42
.csharpCopy codeint intValue = 42;
- Boxing Operation: When you want to box this
int
value, you assign it to a variable of a reference type, such asobject
. This process involves creating a new object on the heap and copying the value of theint
into that object. TheboxedValue
variable now refers to this newly created object. At this point,boxedValue
contains a reference to an object on the heap, which holds a copy of theint
value42
.
Unboxing:
- Retrieve the Boxed Object: To unbox the value, you first need a reference to the boxed object. In our example,
boxedValue
contains the reference to the boxedint
.Assuming boxedValue still refers to the boxed int
- Unboxing Operation: To retrieve the original
int
value from the boxed object, you explicitly cast theboxedValue
variable back to its original value type (int
in this case).csharpCopy codeint unboxedValue = (int)boxedValue; // Unboxing operation
The unboxing operation checks if the boxed object is compatible with the specified target value type (int
in this case). If it is, it extracts the value from the boxed object and assigns it to theunboxedValue
variable.
Input/ Output
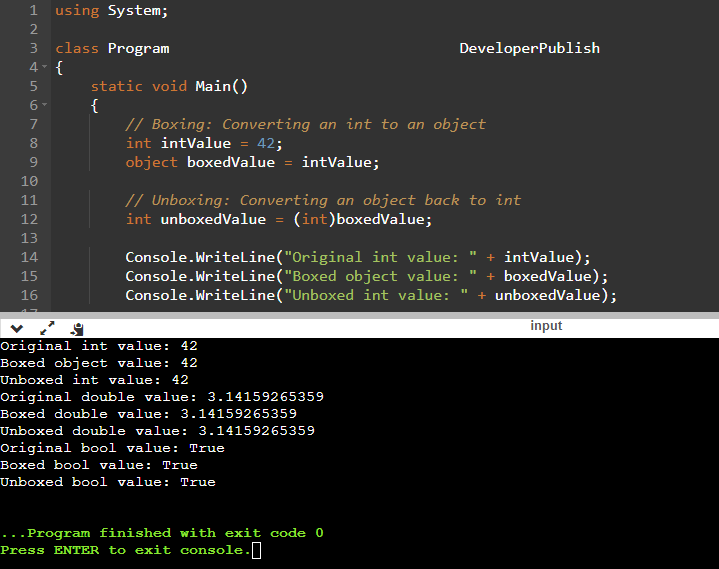