In this example, we’ll create a C# program that prints odd numbers within a specified range, showcasing the language’s capabilities in implementing loops and conditional statements.
Problem Statement
Write a C# program to print all the odd numbers within a given range. The program should take two integer inputs, representing the start and end of the range, and then display all the odd numbers within that range.
C# Program to Print Odd Numbers in a Given Range
using System; class Program { static void Main() { Console.Write("Enter the starting number: "); int start = Convert.ToInt32(Console.ReadLine()); Console.Write("Enter the ending number: "); int end = Convert.ToInt32(Console.ReadLine()); Console.WriteLine("Odd numbers in the range from {0} to {1}:", start, end); PrintOddNumbersInRange(start, end); } static void PrintOddNumbersInRange(int start, int end) { for (int i = start; i <= end; i++) { if (i % 2 != 0) { Console.WriteLine(i); } } } }
Input / Output
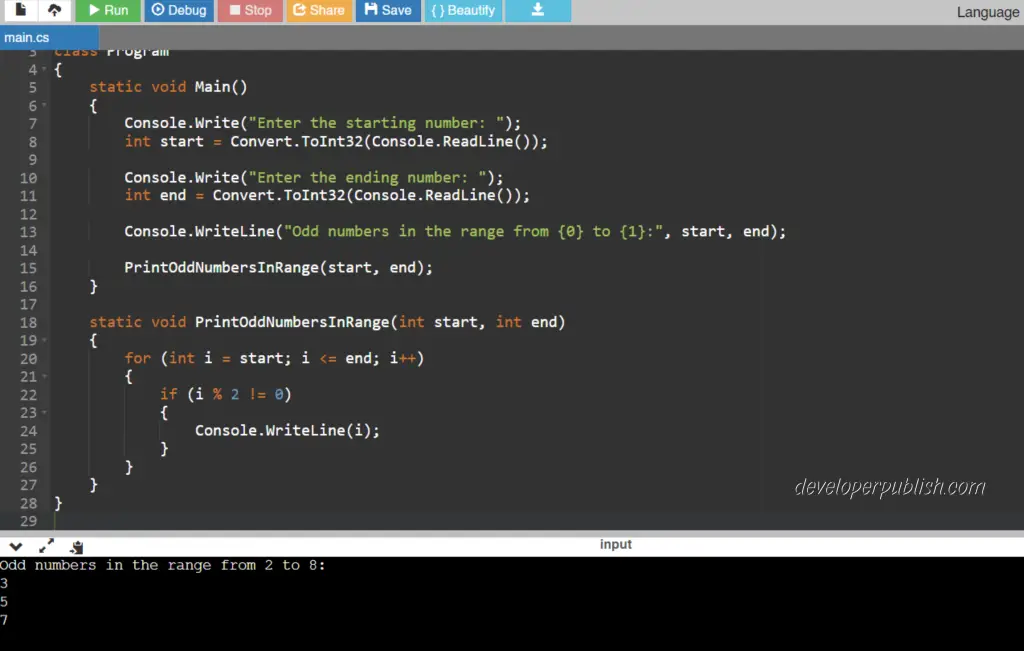