In computer programming, bitwise operations are frequently used to manipulate individual bits in a binary representation of data. One common task is to determine whether a specific bit at a given position is set (1) or not (0). In this post, we will explore a C program that checks whether the nth bit of a number is set or not, using bitwise operations.
Problem statement
Given an integer number ‘num’ and a positive integer ‘n’, we need to determine whether the nth bit of ‘num’ is set (1) or not (0). The program should output the result based on the evaluation.
C Program to Check whether nth Bit is Set or not
#include <stdio.h> int isNthBitSet(int num, int n) { // Shifting 1 by n positions to the left int mask = 1 << n; // Bitwise AND operation to check if the nth bit is set or not if (num & mask) return 1; // nth bit is set (1) else return 0; // nth bit is not set (0) } int main() { int num, n; printf("Enter a number: "); scanf("%d", &num); printf("Enter the position (n) of the bit to check: "); scanf("%d", &n); int result = isNthBitSet(num, n); printf("The %dth bit of %d is %s.\n", n, num, result ? "set" : "not set"); return 0; }
Input/Output
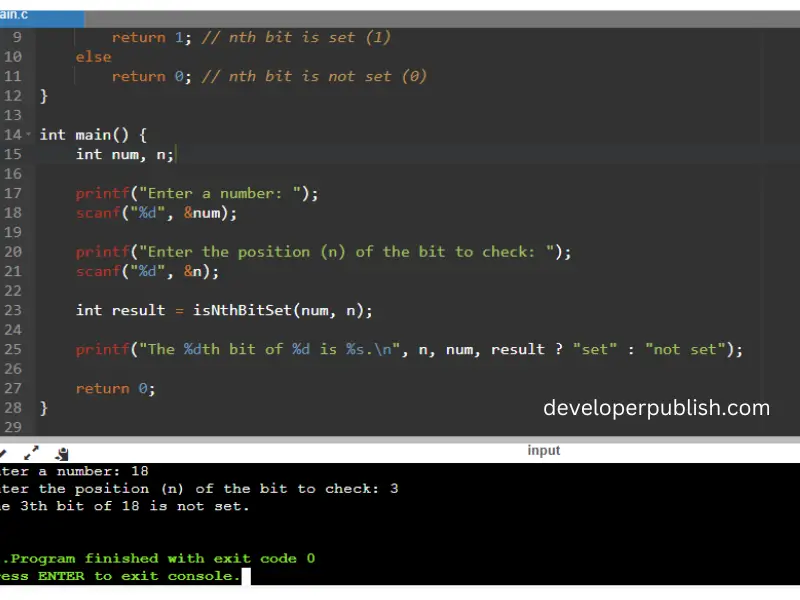