In this program, we will learn how to create a file in C and store information in it. File handling is an essential concept in programming, allowing us to read from and write to files on the computer’s storage.
Problem statement
We need to create a C program that asks the user to enter their name and age. The program will then create a file named “information.txt” and store the entered information in it.
C Program to Create a File and Store Information
#include <stdio.h> #include <stdlib.h> int main() { FILE *file; char name[50]; int age; // Introduction printf("File Creation and Information Storage\n"); printf("------------------------------------\n"); // Problem statement printf("Enter your name: "); fgets(name, sizeof(name), stdin); printf("Enter your age: "); scanf("%d", &age); // Open the file in write mode file = fopen("information.txt", "w"); if (file == NULL) { printf("Failed to create the file.\n"); return 1; } // Store information in the file fprintf(file, "Name: %s", name); fprintf(file, "Age: %d", age); // Close the file fclose(file); printf("Information stored successfully in 'information.txt' file.\n"); return 0; }
Input\Output
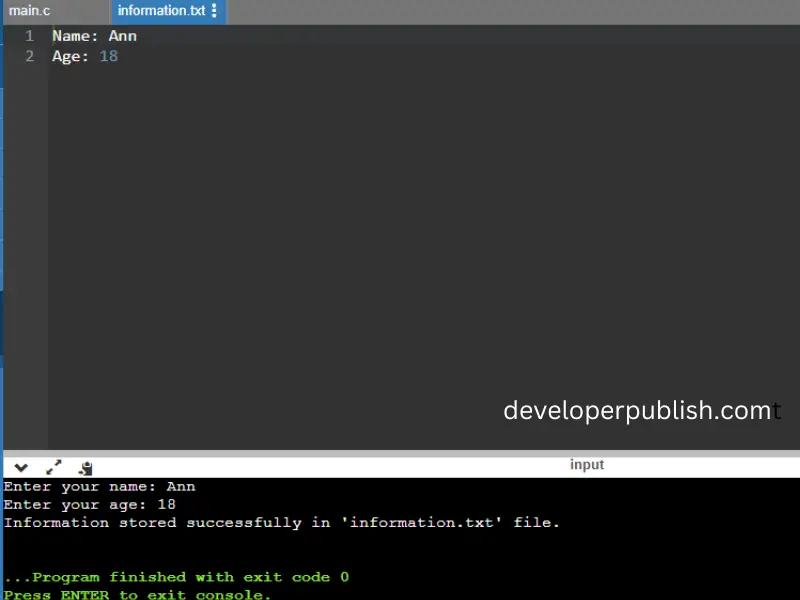