C# Compiler Error
CS0539 – ‘member’ in explicit interface declaration is not a member of interface
Reason for the Error
You’ll get this error in your C# code when you are trying to explicitly declare an interface member that does not exist.
For example, let’s try to compile the below C# code snippet.
using System; namespace DeveloperPublishConsoleCore { interface IEmployee { void GetDetails(); } public class TempEmployee { public void SetDate() { } } class PermanentEmploee : IEmployee { void IEmployee.GetDetails() { } void IEmployee.SetDate() { } } internal class Program { static void Main(string[] args) { Console.WriteLine("DeveloperPublish Hello World!"); } } }
In the above example, we are trying to explictly declare an interface member SetDate() in the PermanentEmploee that doesnot exist in the interface IEmployee. This will trigger the errorcode CS0539.
Error CS0539 ‘PermanentEmploee.SetDate()’ in explicit interface declaration is not found among members of the interface that can be implemented DeveloperPublishConsoleCore C:\Users\senth\source\repos\DeveloperPublishConsoleCore\DeveloperPublishConsoleCore\Program.cs 23 Active
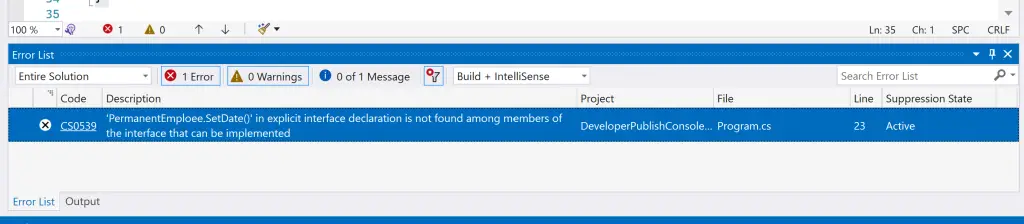
Solution
To fix this error in your C# code, you will need to delete the declaration or else update the class to refer to a valid interface member.
using System; namespace DeveloperPublishConsoleCore { interface IEmployee { void GetDetails(); } public class TempEmployee { public void SetDate() { } } class PermanentEmploee : IEmployee { void IEmployee.GetDetails() { } } internal class Program { static void Main(string[] args) { Console.WriteLine("DeveloperPublish Hello World!"); } } }