C# Compiler Error
CS0538 – ‘name’ in explicit interface declaration is not an interface
Reason for the Error
You’ll get this error in your C# code when you are trying to explicitly declare an interface but an interface was not specified.
For example, let’s try to compile the below C# code snippet.
using System; namespace DeveloperPublishConsoleCore { interface IEmployee { void GetDetails(); } public class TempEmployee { public void SetDate() { } } class PermanentEmploee : IEmployee { void IEmployee.GetDetails() { } // The below results in the error code CS0538 void TempEmployee.SetDate() { } } internal class Program { static void Main(string[] args) { Console.WriteLine("DeveloperPublish Hello World!"); } } }
In the above example, we are trying to explictly declare an interface in the PermanentEmploee but using the classname instead. This will trigger the errorcode CS0538.
Error CS0538 ‘TempEmployee’ in explicit interface declaration is not an interface DeveloperPublishConsoleCore C:\Users\senth\source\repos\DeveloperPublishConsoleCore\DeveloperPublishConsoleCore\Program.cs 23 Active
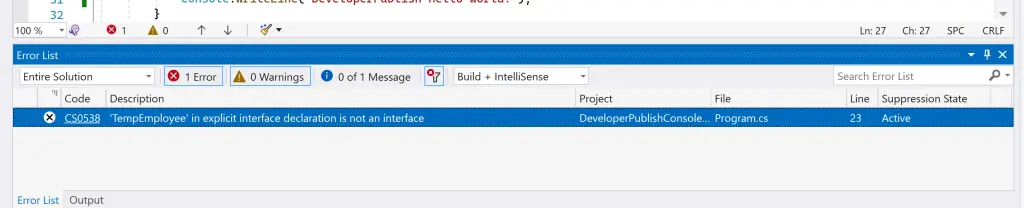
Solution
In C#, an explicit interface implementation is a member of the class that can only be called through the specified interface name, You will need to specify it with the name of the interface followed by period and then by the name of the method from the interface.
In the above code snippet, you’ll need to remove the TempEmployee.SetDate() call.