C# Compiler Error
CS0535 – ‘class’ does not implement interface member ‘member’
Reason for the Error
You’ll get this error in your C# code when the class that is derived from the interface does not implement one or more of the interface members.
For example, let’s try to compile the below C# code snippet.
using System; namespace DeveloperPublishConsoleCore { public interface IEmployee { void GetDetails(); } public class Employee : IEmployee { } internal class Program { static void Main(string[] args) { Console.WriteLine("DeveloperPublish Hello World!"); } } }
In the above example, the Employee class derives from the interface IEmployee but doesnot implement the method GetDetails(). This results in the C# error code CS0535.
Error CS0535 ‘Employee’ does not implement interface member ‘IEmployee.GetDetails()’ DeveloperPublishConsoleCore C:\Users\senth\source\repos\DeveloperPublishConsoleCore\DeveloperPublishConsoleCore\Program.cs 10 Active
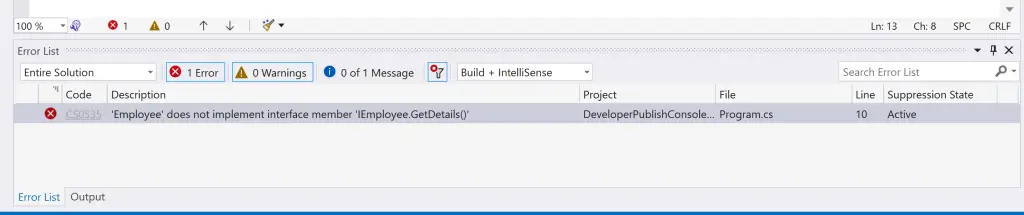
Solution
In C#, it is mandatory for the class to implement all the members of the interface from which it derives. You can fix the above code by implementing the method GetDetails() in the Employee class.
using System; namespace DeveloperPublishConsoleCore { public interface IEmployee { void GetDetails(); } public class Employee : IEmployee { public void GetDetails() { // Write your Logic here } } internal class Program { static void Main(string[] args) { Console.WriteLine("DeveloperPublish Hello World!"); } } }