C# Compiler Error
CS0533 – ‘derived-class member’ hides inherited abstract member ‘base-class member’
Reason for the Error
You’ll get this error in your C# code when your base class method is hidden.
For example, let’s try to compile the below C# code snippet.
using System; namespace DeveloperPublishConsoleCore { abstract public class ParentClass { abstract public void Method1(); } abstract public class ChildClass : ParentClass { new abstract public void Method1(); } internal class Program { static void Main(string[] args) { Console.WriteLine("DeveloperPublish Hello World!"); } } }
The above program has a ParentClass with an abstract method Method1. The ChildClass inherits from the ParentClass but also has a method Method1 defined with the new keyword. This hides the base class’s method Method1 and C# compiler will throw the error code CS0533.
Error CS0533 ‘ChildClass.Method1()’ hides inherited abstract member ‘ParentClass.Method1()’ DeveloperPublishConsoleCore C:\Users\senth\source\repos\DeveloperPublishConsoleCore\DeveloperPublishConsoleCore\Program.cs 12 Active
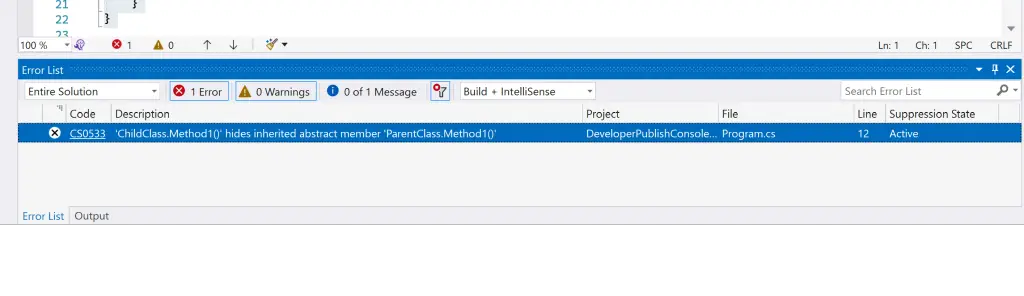
Solution
To fix this error, you’ll need to override the method in the inherited class instead of hiding the base class method.
using System; namespace DeveloperPublishConsoleCore { abstract public class ParentClass { abstract public void Method1(); } abstract public class ChildClass : ParentClass { // This line replaced with override to fix the error code CS0533 override abstract public void Method1(); } internal class Program { static void Main(string[] args) { Console.WriteLine("DeveloperPublish Hello World!"); } } }