C# Compiler Error
CS0506 – ‘function1’ : cannot override inherited member ‘function2’ because it is not marked “virtual”, “abstract”, or “override”
Reason for the Error
You’ll get this error in your C# code when you attempt to override a member that is not explicitly marked as virtual, abstract, or override in the base class.
For example, let’s try to compile the below C# code snippet.
using System; namespace DeveloperPublishNamespace { public class BaseClass { public int Id { get; set; } } public class ChildClass : BaseClass { public override int Id { get; set; } } class Program { static void Main(string[] args) { Console.WriteLine("No Error"); } } }
You’ll receive the error code CS0505 because the C# compiler has detected that you are overriding a property Id that is not marked as either abstract or virtual in the base class.
Error CS0506 ‘ChildClass.Id’: cannot override inherited member ‘BaseClass.Id’ because it is not marked virtual, abstract, or override DeveloperPublish C:\Users\Senthil\source\repos\ConsoleApp4\ConsoleApp4\Program.cs 12 Active
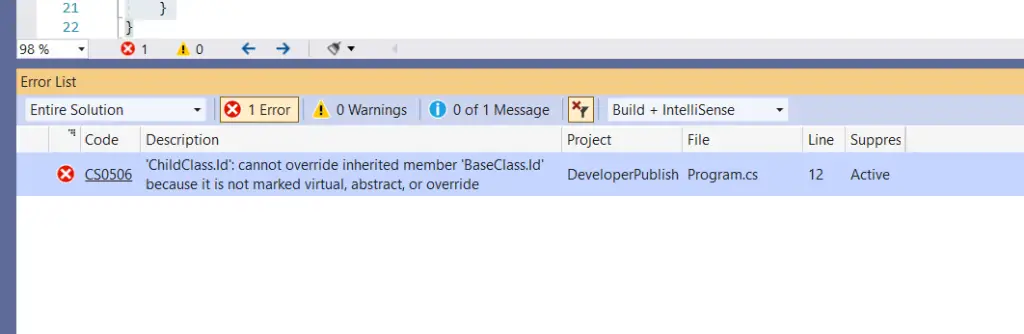
Solution
You can fix this error in your C# program by marking the member as virtual or abstract in the base class as shown below.
using System; namespace DeveloperPublishNamespace { public class BaseClass { public virtual int Id { get; set; } } public class ChildClass : BaseClass { // This returns the error CS0505 public override int Id { get; set; } } class Program { static void Main(string[] args) { Console.WriteLine("No Error"); } } }