C# Compiler Error
CS0505 – ‘member1’: cannot override because ‘member2’ is not a function
Reason for the Error
You’ll get this error in your C# code when you attempted to override a non-method in a base class.
For example, let’s try to compile the below C# code snippet.
using System; namespace DeveloperPublishNamespace { public class BaseClass { public virtual int Id { get; set; } } public class ChildClass : BaseClass { // This returns the error CS0505 public override int Id(){ return 0; } } class Program { static void Main(string[] args) { Console.WriteLine("No Error"); } } }
You’ll receive the error code CS0505 because the C# compiler has detected that you have a property defined in the base class but you are trying to override a function or method with the same name.
Error CS0505 ‘ChildClass.Id()’: cannot override because ‘BaseClass.Id’ is not a function C:\Users\SenthilBalu\source\repos\ConsoleApp4\ConsoleApp4\Program.cs 12 Active
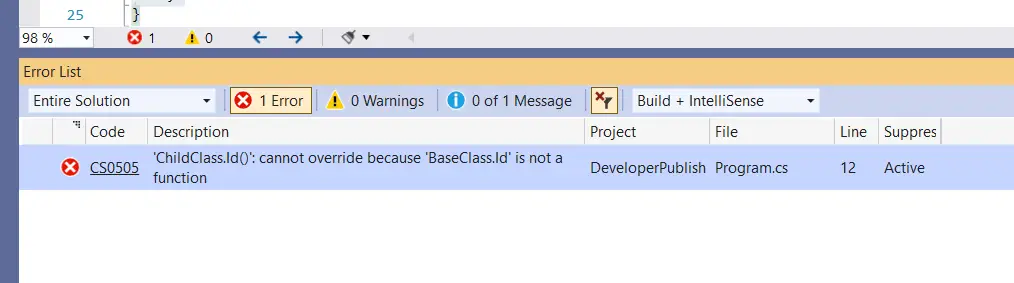
Solution
In the C# programming language, the overrides must match the exact member type. You can fix this error in your C# program by ensuring that the override matches the member type. In the above example, override the property.
using System; namespace DeveloperPublishNamespace { public class BaseClass { public virtual int Id { get; set; } } public class ChildClass : BaseClass { // This returns the error CS0505 public override int Id { get; set; } } class Program { static void Main(string[] args) { Console.WriteLine("No Error"); } } }