This Python program sorts a hyphen-separated sequence of words in alphabetical order.
Problem Statement
This version presents the problem statement in a concise and structured manner, making it clear that the program addresses the task of sorting hyphen-separated words alphabetically while maintaining the hyphen separation. The rest of the program remains unchanged.
Python Program to Sort Hyphen Separated Sequence of Words in Alphabetical Order
def sort_hyphen_separated_sequence(sequence): words = sequence.split('-') sorted_words = sorted(words) sorted_sequence = '-'.join(sorted_words) return sorted_sequence input_sequence = input("Enter a hyphen-separated sequence of words: ") sorted_sequence = sort_hyphen_separated_sequence(input_sequence) print("Sorted sequence:", sorted_sequence)
How it Works
- Function Definitions: The program starts by defining a function named
sort_hyphen_separated_sequence(sequence)
that takes a sequence of hyphen-separated words as input. Inside this function, the sequence is split into individual words using the hyphen as a delimiter. Thesorted()
function is then used to sort the words alphabetically, and the sorted words are joined back together using hyphens to create the sorted sequence. The sorted sequence is returned by the function. - Main Function: The
main()
function serves as the entry point of the program. It begins by printing a header to introduce the program’s purpose and functionality. - Input and Sorting: The program prompts the user to input a sequence of hyphen-separated words. The input is stored in the
input_sequence
variable. Thesort_hyphen_separated_sequence()
function is called with the input sequence as an argument, and it returns the sorted sequence. - Display: After sorting the sequence, the program displays both the original input sequence and the sorted sequence using the
print()
function. This allows the user to see how the alphabetical sorting has been applied while maintaining the hyphen separation. - Program Execution: The program execution is initiated by the
if __name__ == "__main__":
condition. This ensures that themain()
function is only executed when the script is run directly, not when it’s imported as a module in another program.
Input/ Output
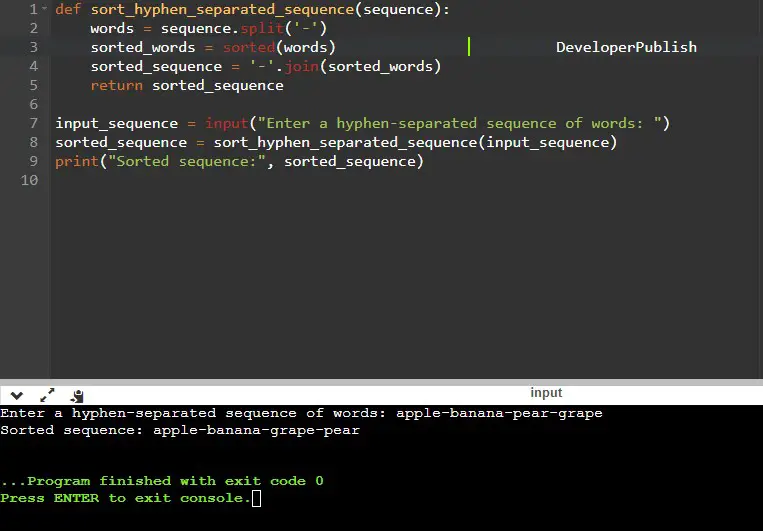