This Python program counts the frequency of each word in a string using a dictionary.ch.
Problem Statement
Write a Python program to count the frequency of each word in a given string. Your program should use a dictionary to store the word frequencies and provide the count for each unique word in the string. The program should be case-insensitive and should not consider common punctuation marks while identifying words.
Python Program to Count the Frequency of Each Word in a String using Dictionary
def count_word_frequency(input_string): # Removing punctuation and converting the string to lowercase cleaned_string = input_string.lower().replace('.', '').replace(',', '').replace('!', '').replace('?', '') # Splitting the string into words words = cleaned_string.split() # Creating an empty dictionary to store word frequencies word_frequency = {} # Counting word frequencies for word in words: if word in word_frequency: word_frequency[word] += 1 else: word_frequency[word] = 1 return word_frequency # Input string input_string = "This is a sample string, and this is a simple program. Is this a sample or a simple string?" # Counting word frequency frequency_dict = count_word_frequency(input_string) # Printing word frequencies for word, frequency in frequency_dict.items(): print(f"'{word}': {frequency}")
How it Works
- The
count_word_frequency
function takes an input string as an argument. - The input string is first cleaned by converting it to lowercase and removing common punctuation marks (periods, commas, exclamation marks, and question marks) using the
replace
method. - The cleaned string is then split into individual words using the
split
method, creating a list calledwords
. - An empty dictionary called
word_frequency
is created to store the word frequencies. - The program iterates through each word in the
words
list. If the word is already present in theword_frequency
dictionary, its count is incremented. Otherwise, a new entry is added to the dictionary with the word as the key and the value set to 1. - Once all the words have been processed, the
word_frequency
dictionary containing the word frequencies is returned. - The program then uses the
input_string
to count word frequencies by calling thecount_word_frequency
function. - The word frequencies are printed out using a loop that iterates over the items in the
frequency_dict
dictionary. Each word and its corresponding frequency are printed in the specified format.
Input/ Output
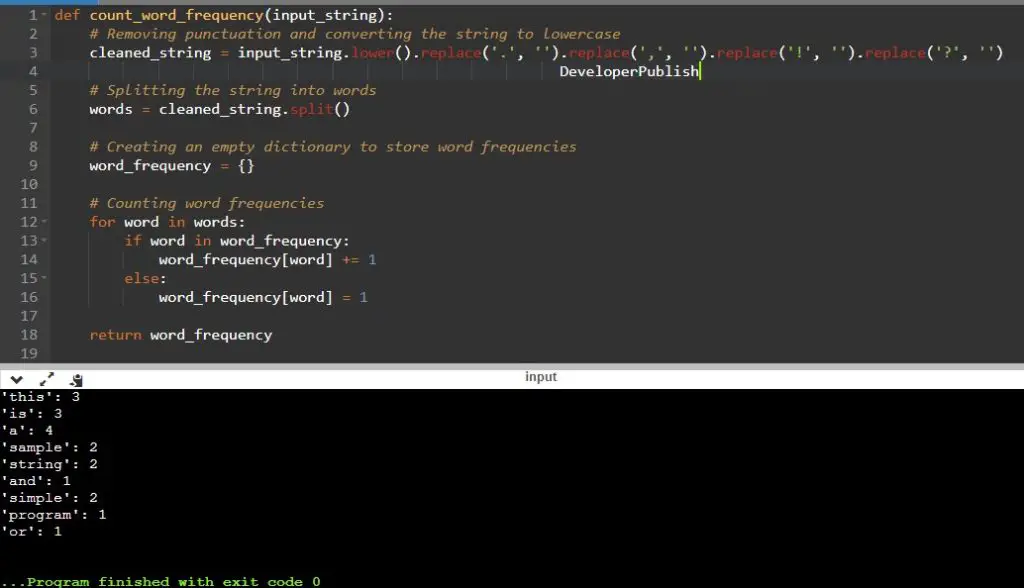