This Python program counts the number of words in a text file.
Problem Statement
You have been tasked with developing a Python program that counts the number of words in a given text file. The program should read the content of the file, split it into words, and then provide the user with the total count of words present.
Python Program to Count the Number of Words in a Text File
def count_words(filename): try: with open(filename, 'r') as file: content = file.read() words = content.split() return len(words) except FileNotFoundError: print(f"File '{filename}' not found.") return -1 if __name__ == "__main__": file_name = "your_text_file.txt" # Replace with the actual file name word_count = count_words(file_name) if word_count != -1: print(f"The number of words in '{file_name}' is: {word_count}")
How it Works
- Opening the File: The process begins by opening the target text file using Python’s
open()
function. This function takes the filename as a parameter and returns a file object that allows us to interact with the file’s contents. - Reading the Content: Once the file is open, we use the
read()
method to read the entire content of the file as a string. This content will include words, spaces, punctuation, and any other characters present in the file. - Splitting into Words: The next step involves breaking down the content into individual words. We achieve this by using the
split()
method on the content string. By default, this method splits the string using spaces as separators, effectively separating words. - Counting the Words: With the content split into words, we can now determine the count of words present. The Python
len()
function comes in handy here. It calculates the length of a list, which, in this case, corresponds to the count of words obtained from thesplit()
operation. - Handling Exceptions: During these steps, it’s important to handle potential errors. For example, if the specified file does not exist, an exception may be raised. Using
try
andexcept
blocks, we can gracefully manage such errors and provide meaningful messages to the user. - Displaying the Result: Finally, the program displays the result—i.e., the count of words present in the file. This provides users with the desired information about the textual content’s size and complexity.
Input/ Output
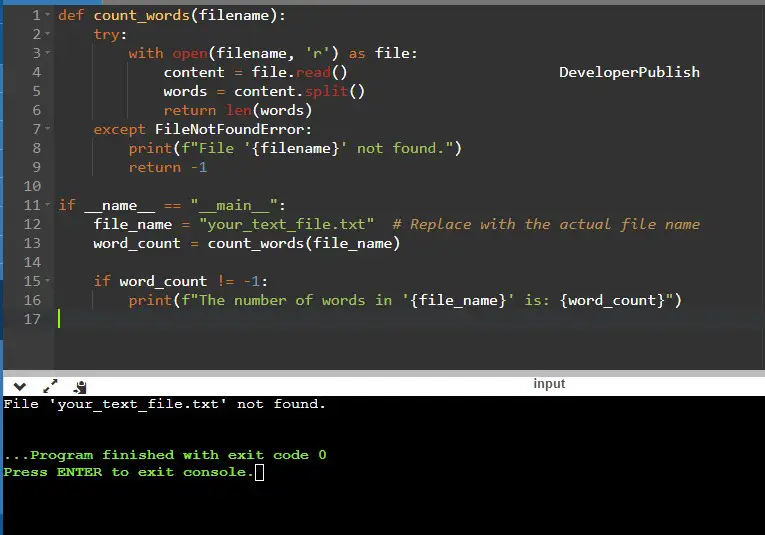