This Python program counts the occurrences of each word in a string and prints the word counts.
Problem Statement
You are tasked with creating a Python program that counts the occurrences of each word in a given input string. Your program should take the input string, process it, and then display the words along with their respective counts.
Python Program to Count the Occurrences of a Word in a Text File
def count_word_occurrences(input_string): # Convert the input string to lowercase and split it into words words = input_string.lower().split() # Create an empty dictionary to store word counts word_count = {} # Loop through the words and update the word counts for word in words: if word in word_count: word_count[word] += 1 else: word_count[word] = 1 return word_count # Get input from the user input_string = input("Enter a string: ") # Call the function to count word occurrences word_occurrences = count_word_occurrences(input_string) # Display the word occurrences for word, count in word_occurrences.items(): print(f"'{word}' occurs {count} times.")
How it Works
- The program defines a function
count_word_occurrences(input_string)
to count word occurrences in a given input string. - The input string is converted to lowercase and split into words using
lower()
andsplit()
functions, creating a list of words. - An empty dictionary
word_count
is created to store word occurrences. - The program loops through each word in the list:
- If the word is already in
word_count
, its count is incremented. - If the word is not in
word_count
, a new entry is created with a count of 1.
- If the word is already in
- The
count_word_occurrences
function returns theword_count
dictionary. - The program gets user input, calls the function, and stores the result.
- It iterates through the dictionary and prints each word along with its count.
Input/ Output
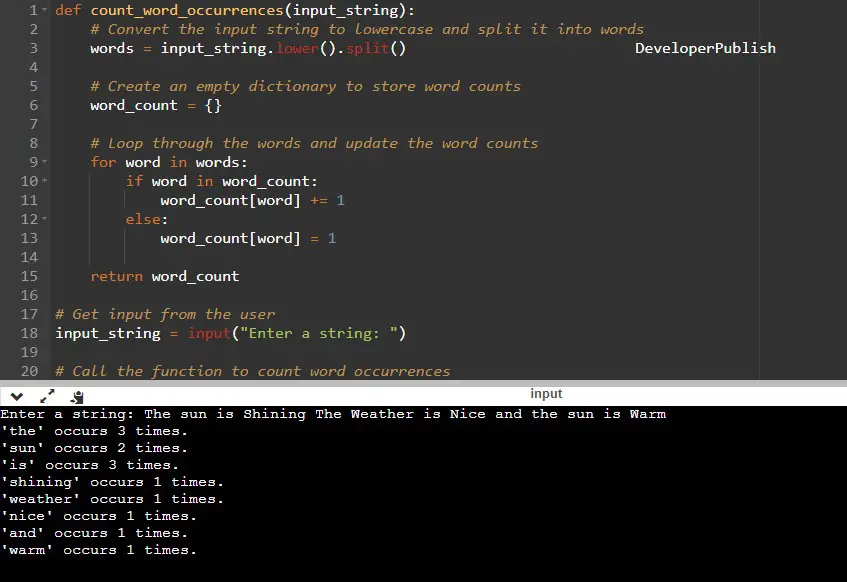