In this Python program, we will create a class called SubsetGenerator
to find all possible distinct subsets of a given set. Subsets are the combinations of elements from the original set, and we will implement a method to generate these subsets.
Problem statement
Given a set, we need to create a class that can generate all distinct subsets of the set, including the empty set and the set itself.
Python Program to Create a Class and Get All Possible Distinct Subsets from a Set
class SubsetGenerator: def __init__(self, original_set): self.original_set = original_set self.subsets = [] def generate_subsets(self): self._generate_subsets_recursive([], 0) return self.subsets def _generate_subsets_recursive(self, current_subset, start_index): self.subsets.append(current_subset[:]) # Add a copy of the current subset for i in range(start_index, len(self.original_set)): current_subset.append(self.original_set[i]) self._generate_subsets_recursive(current_subset, i + 1) current_subset.pop() def main(): input_set = input("Enter elements of the set separated by spaces: ").split() subset_generator = SubsetGenerator(input_set) subsets = subset_generator.generate_subsets() print("\nAll possible distinct subsets:") for subset in subsets: print(subset) if __name__ == "__main__": main()
How it works
- The program defines a class
SubsetGenerator
with an initializer that takes the original set as input and initializes an empty listsubsets
to store the generated subsets. - The
generate_subsets
method initializes the recursive process to generate subsets and returns the list of subsets. - The
_generate_subsets_recursive
method is a helper function that generates subsets recursively. It starts by adding a copy of the current subset to thesubsets
list. - It then iterates through the remaining elements of the original set, recursively adding and removing elements to generate subsets.
- The
main
function takes user input for the set elements, creates an instance ofSubsetGenerator
, and generates subsets. - The program finally prints all the generated subsets.
Input / Output
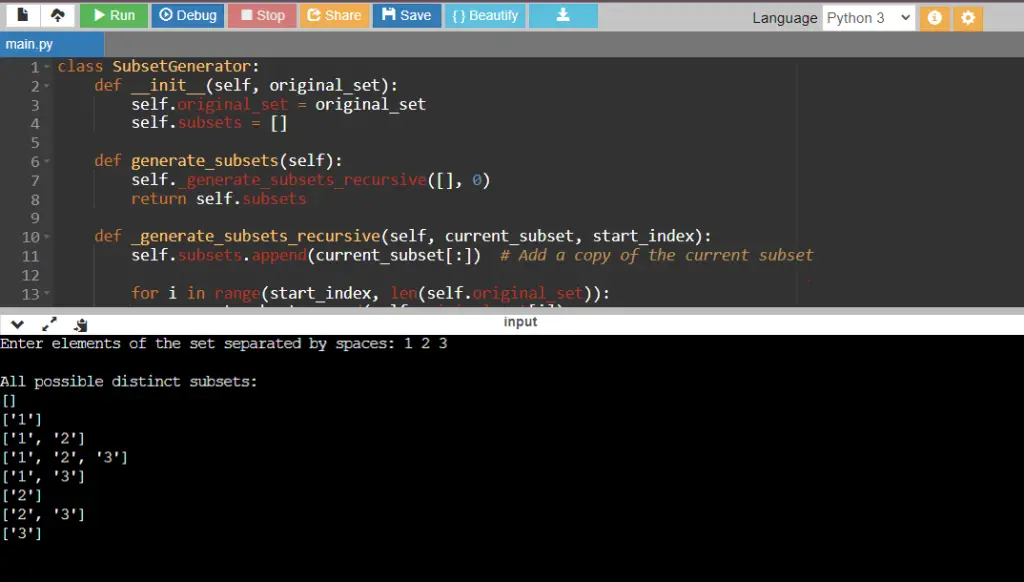