This Python program is designed to count the number of lines in a text file. It’s a simple yet useful script that can help you quickly determine how many lines are present in a given text file. The program works by opening the specified file, reading its contents line by line, and keeping track of the number of lines encountered.
Problem Statement
You are tasked with creating a Python program that counts the number of lines in a given text file. Your program should be able to handle cases where the specified file does not exist or there is an issue while reading the file.
Python Program to Count the Number of Lines in Text File
def count_lines(filename): try: with open(filename, 'r') as file: line_count = 0 for line in file: line_count += 1 return line_count except FileNotFoundError: return -1 # Return -1 to indicate file not found except Exception as e: return -2 # Return -2 to indicate an error file_name = "your_text_file.txt" # Replace with your file's name line_count = count_lines(file_name) if line_count == -1: print("File not found.") elif line_count == -2: print("An error occurred while reading the file.") else: print(f"Number of lines in the file: {line_count}")
How it Works
- Function Definition:
The program starts by defining a function named count_lines
that takes a filename as an argument. This function will be responsible for counting the lines in the specified text file.
- Function Explanation:
- The
try
block attempts to open the specified file using theopen()
function in read mode ('r'
). Thewith
statement ensures that the file is properly closed after reading. - Inside the
with
block, a variable namedline_count
is initialized to keep track of the number of lines encountered in the file. - The program then enters a loop that iterates through each line in the file. For each line, the
line_count
is incremented by 1. - After reading all lines in the file, the function returns the final value of
line_count
, which represents the total number of lines in the file. - If the specified file is not found (raises a
FileNotFoundError
), the function returns-1
to indicate this issue. - If any other error occurs while reading the file, the function returns
-2
to indicate the error.
- The
- Main Part of the Program:
In the main part of the program, you need to replace "your_text_file.txt"
with the actual filename you want to analyze. The count_lines
function is called with this filename, and the returned value is stored in the line_count
variable.
- Output Handling:
Depending on the value of line_count
, the program prints different outputs.
- If
line_count
is-1
, it means the file was not found, so the program prints “File not found.” - If
line_count
is-2
, it indicates an error occurred during file reading, so the program prints “An error occurred while reading the file.” - Otherwise, the program prints the actual number of lines in the file using an f-string.
- Usage:
- Replace
"your_text_file.txt"
with the actual path or name of the text file you want to analyze. - Run the Python script.
- Observe the output to see the number of lines in the specified text file.
- Replace
Input/ Output
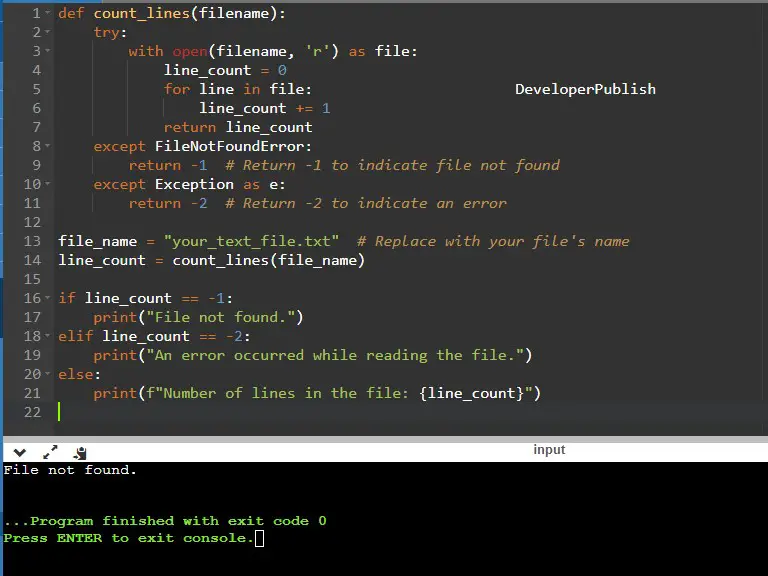