This Python program finds and displays letters that are not common in two strings.
Problem Statement
You are tasked with writing a Python program to determine and display the letters that are not common between two input strings.
Python Program that Displays Letters that are not Common in Two Strings
def uncommon_letters(str1, str2): set1 = set(str1) set2 = set(str2) uncommon = set1.symmetric_difference(set2) return uncommon string1 = input("Enter the first string: ") string2 = input("Enter the second string: ") result = uncommon_letters(string1, string2) print("Letters that are not common:", ', '.join(result))
How it Works
- Function Definition:pythonCopy code
def uncommon_letters(str1, str2):
We define a function calleduncommon_letters
that takes two string argumentsstr1
andstr2
. - Creating Sets:pythonCopy code
set1 = set(str1) set2 = set(str2)
We convert each input string into sets using theset()
function. A set is an unordered collection of unique elements. This helps us to efficiently find the unique letters in each string. - Finding Uncommon Letters:pythonCopy code
uncommon = set1.symmetric_difference(set2)
Thesymmetric_difference()
method of sets returns a new set containing all elements that are in either of the sets, but not in their intersection. In other words, it gives us the unique elements from both sets. - Returning Uncommon Letters:pythonCopy code
return uncommon
Theuncommon_letters
function returns the set of letters that are not common between the two input strings. - Taking User Input:pythonCopy code
string1 = input("Enter the first string: ") string2 = input("Enter the second string: ")
The program prompts the user to enter two strings. - Calling the Function and Getting Results:pythonCopy code
result = uncommon_letters(string1, string2)
Theuncommon_letters
function is called with the input strings, and the result is stored in theresult
variable. - Displaying Output:pythonCopy code
print("Letters that are not common:", ', '.join(result))
The program prints the unique letters using thejoin()
function to create a comma-separated string from the set.
For example, if you enter "hello"
and "world"
as input, the program converts them into sets {'h', 'e', 'l', 'o'}
and {'w', 'o', 'r', 'l', 'd'}
respectively. The symmetric difference between these sets gives {'h', 'e', 'd', 'r', 'w'}
, which are the letters not common between the two strings. The program then prints this set of letters as the output.
Input/ Output
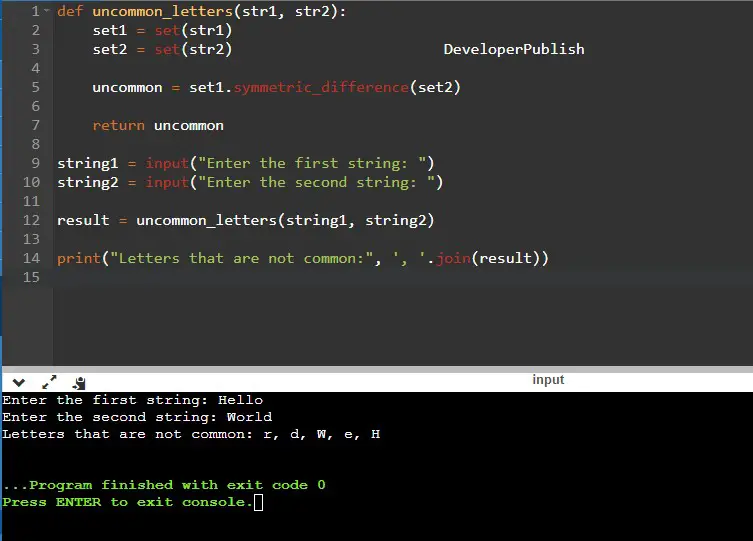