In the realm of programming, string manipulation is a fundamental skill. Counting the number of vowels in a string is a common programming task that helps to enhance your understanding of basic string operations and loops in Python. This program aims to demonstrate how to accomplish this task efficiently.
Problem Statement
Write a Python program that takes a string as input and calculates the total number of vowels present in the given string. The program should consider both uppercase and lowercase vowels.
Python Program to Count the Number of Vowels in a String
def count_vowels(string): vowels = "aeiouAEIOU" # List of vowels, both lowercase and uppercase vowel_count = 0 for char in string: if char in vowels: vowel_count += 1 return vowel_count # Input input_string = input("Enter a string: ") # Counting vowels vowel_count = count_vowels(input_string) # Output print("The number of vowels in the given string:", vowel_count)
Input / Output
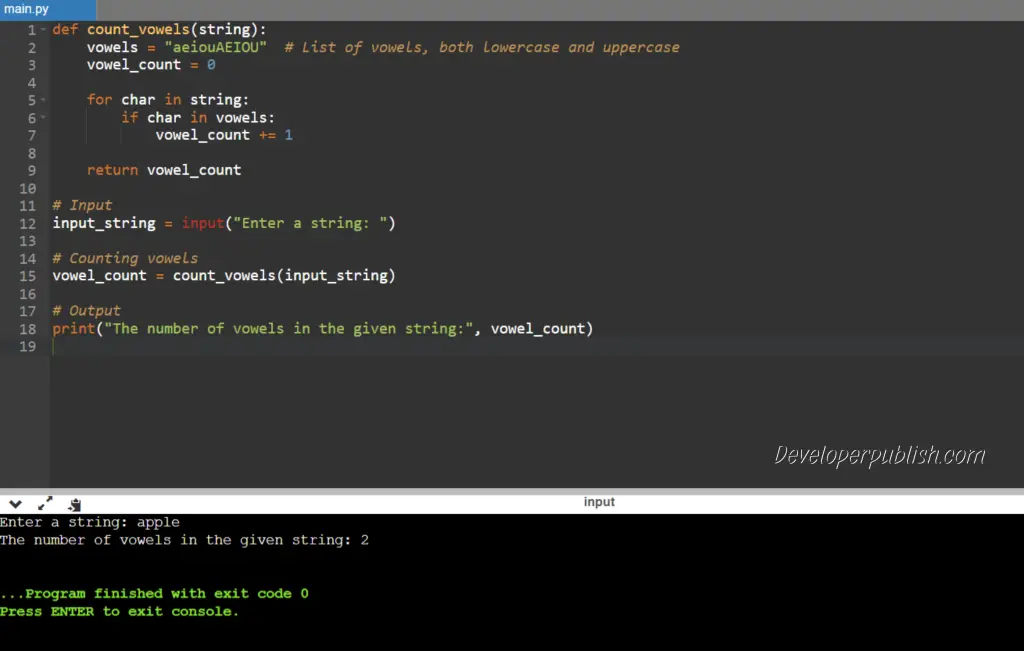