Dictionaries are versatile data structures in Python that allow you to map keys to corresponding values. This program demonstrates how to create a Python program that constructs a dictionary where the keys are the first characters of words, and the values are lists of words that start with the corresponding character.
Problem Statement
Write a Python program that takes a list of words as input and constructs a dictionary with keys as the first characters of words and values as lists of words that start with the corresponding character.
Python Program to Create a Dictionary with Key as First Character and Value as Words Starting with that Character
def create_char_dict(word_list): char_dict = {} for word in word_list: if word[0] in char_dict: char_dict[word[0]].append(word) else: char_dict[word[0]] = [word] return char_dict # Input words = input("Enter a list of words (comma-separated): ").split(',') # Creating the dictionary result_dict = create_char_dict(words) # Output print("Dictionary with words grouped by first character:") for key, value in result_dict.items(): print(f"'{key}': {value}")
Input / Output
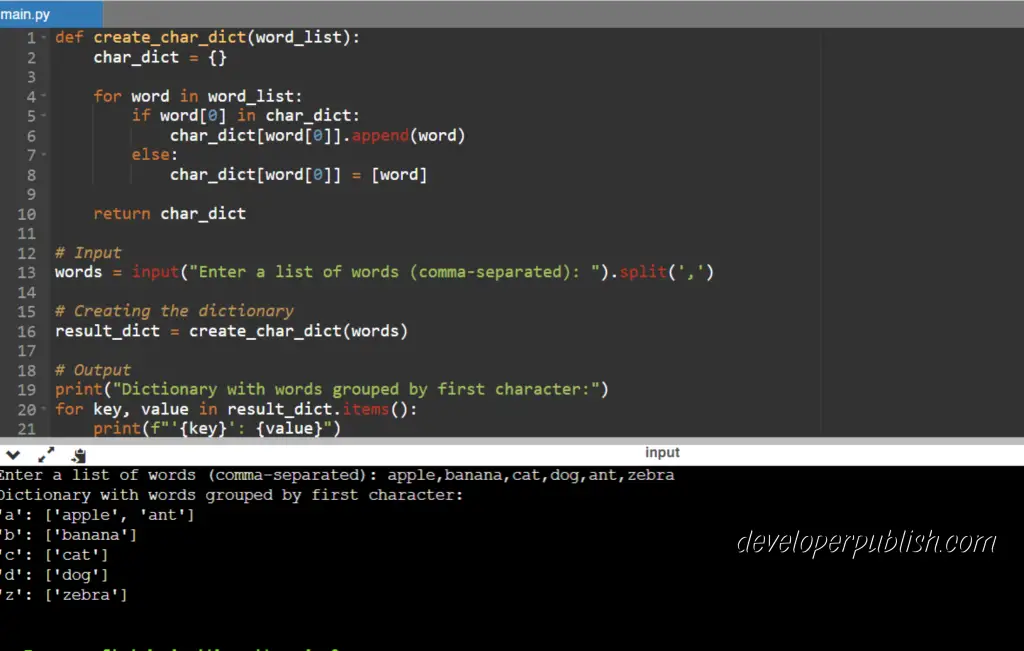