String comparison and manipulation are vital aspects of programming. This program focuses on demonstrating how to create a Python program that finds and prints all the letters that are present in both given strings.
Problem Statement
Write a Python program that takes two strings as input and prints all the letters that are present in both strings, without any duplicates.
Python Program to Print Letters Present in Both Strings
def common_letters(string1, string2): set1 = set(string1) set2 = set(string2) common_chars = set1.intersection(set2) return common_chars # Input string1 = input("Enter the first string: ") string2 = input("Enter the second string: ") # Finding common letters common_chars = common_letters(string1, string2) # Printing common letters if common_chars: print("Common letters:", ', '.join(sorted(common_chars))) else: print("No common letters found.")
Input / Output
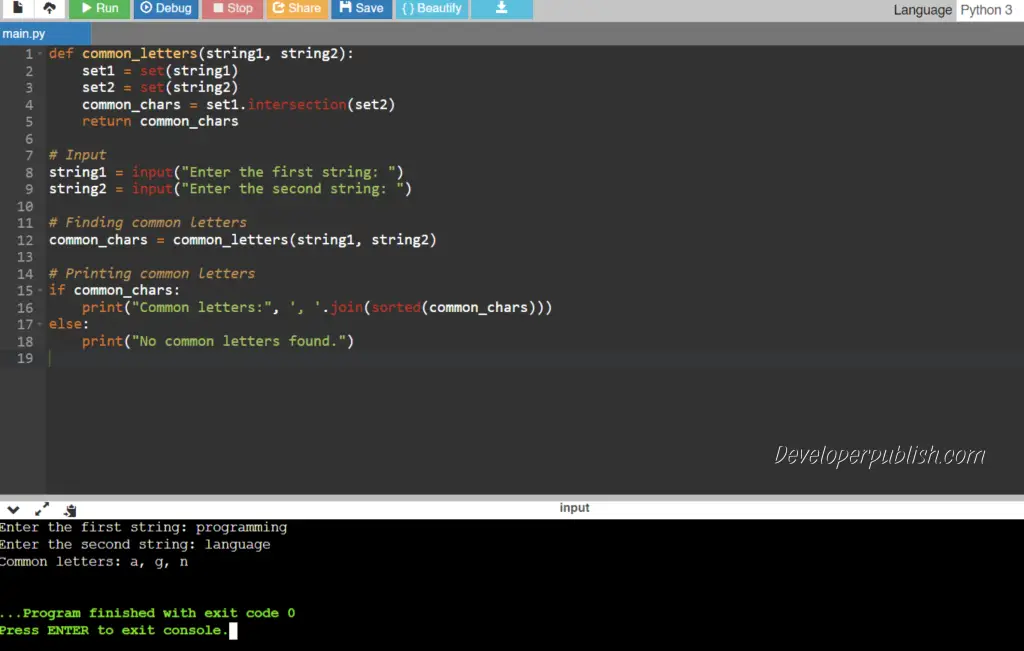