String manipulation is a fundamental skill in programming, and one common task is to determine whether a substring exists within a given string. This program demonstrates how to create a Python program to accomplish this task efficiently using built-in string methods.
Problem Statement
Write a Python program that takes a main string and a substring as input and checks whether the given substring is present within the main string.
Python Program to Check if a Substring is Present in a Given String
def is_substring_present(main_string, substring): if substring in main_string: return True else: return False # Input main_string = input("Enter the main string: ") substring = input("Enter the substring to check: ") # Checking for substring presence if is_substring_present(main_string, substring): print("Substring is present in the main string.") else: print("Substring is not present in the main string.")
Input / Output
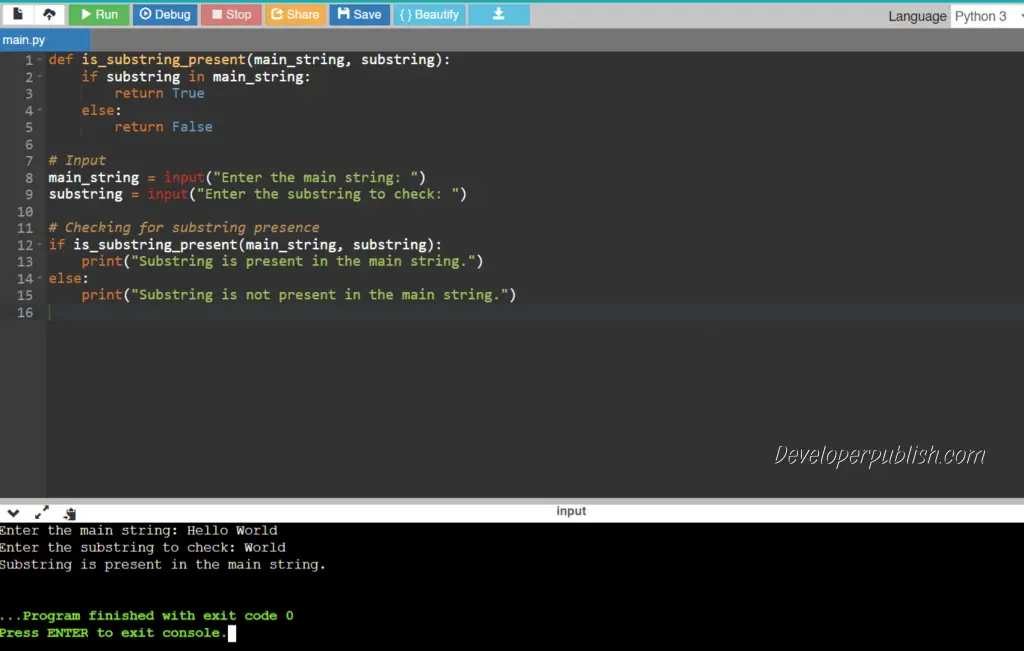