Object-oriented programming (OOP) allows us to create classes that encapsulate data and methods. This program demonstrates how to create a Python program that calculates the area and perimeter of a circle using a class.
Problem Statement
Write a Python program that defines a class for a circle, calculates its area and perimeter, and provides methods to access these values.
Python Program to Find the Area and Perimeter of a Circle using Class
import math class Circle: def __init__(self, radius): self.radius = radius def calculate_area(self): return math.pi * self.radius ** 2 def calculate_perimeter(self): return 2 * math.pi * self.radius # Input radius = float(input("Enter the radius of the circle: ")) # Creating a Circle object circle = Circle(radius) # Calculating area and perimeter area = circle.calculate_area() perimeter = circle.calculate_perimeter() # Output print("Circle with radius", radius) print("Area:", area) print("Perimeter:", perimeter)
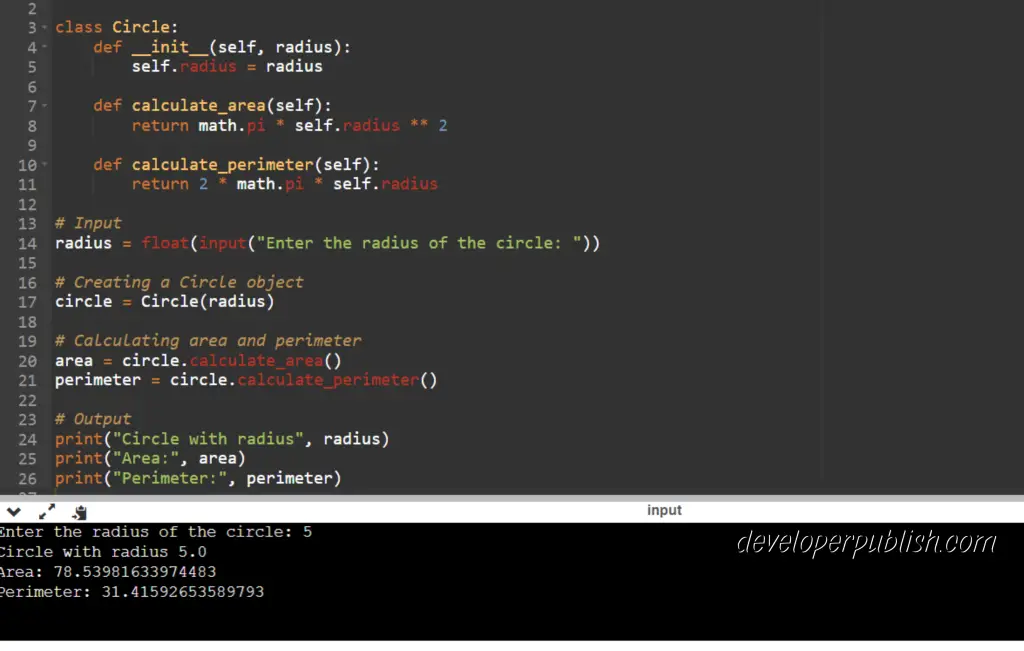