In C programming, it is often necessary to swap the values of two variables. In this program, we will write a C program that prompts the user to enter two numbers, swaps their values, and prints the new values.
Problem Statement
The problem statement is to write a C program that prompts the user to enter two numbers, swaps their values, and prints the new values.
Solution
Here is the C program to swap two numbers:
#include <stdio.h> int main() { int num1, num2, temp; printf("Enter the first number: "); scanf("%d", &num1); printf("Enter the second number: "); scanf("%d", &num2); temp = num1; num1 = num2; num2 = temp; printf("After swapping, the first number is %d and the second number is %d\n", num1, num2); return 0; }
Output
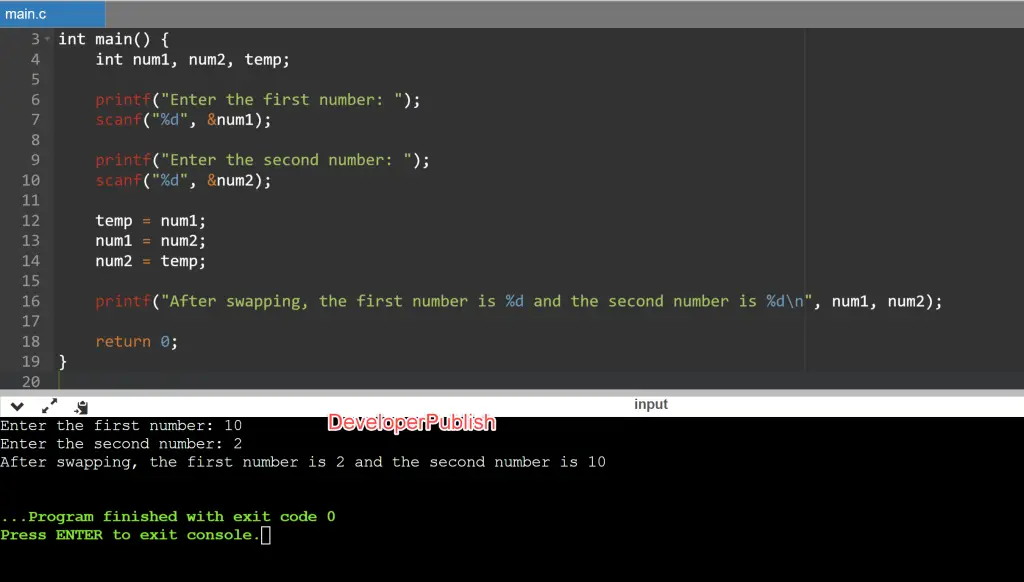
Explanation
First, we include the stdio.h
header file which is used for input and output functions. Then, we define the main()
function as the starting point of our program.
We declare three integer variables – num1
, num2
, and temp
. num1
and num2
are used to store the two numbers entered by the user, and temp
is used to temporarily store the value of num1
while swapping the values.
Next, we prompt the user to enter the first number using the printf()
function. The scanf()
function is used to read in the first number entered by the user. The format specifier %d
is used to specify that the input should be read as an integer.
We then prompt the user to enter the second number using the printf()
function. The scanf()
function is used again to read in the second number entered by the user.
We then use the temp
variable to temporarily store the value of num1
. We assign num2
to num1
and temp
to num2
. This effectively swaps the values of num1
and num2
.
Finally, we print the result using the printf()
function. We use the format specifier %d
to print the values of num1
and num2
after they have been swapped.
Conclusion
In this tutorial, we learned how to write a C program to swap two numbers. We used a temporary variable temp
to store the value of one of the variables while swapping their values. We also used the printf()
function to print the result to the console.