In C programming, it is often necessary to find the sum of the digits of a given number. In this program, we will write a C program that prompts the user to enter a number, calculates the sum of its digits, and prints the result.
Problem Statement
The problem statement is to write a C program that prompts the user to enter a number, calculates the sum of its digits, and prints the result.
Solution
Here is the C program to find the sum of digits of a number:
#include <stdio.h> int main() { int num, sum = 0, remainder; printf("Enter an integer: "); scanf("%d", &num); while(num > 0) { remainder = num % 10; sum += remainder; num /= 10; } printf("The sum of digits is %d\n", sum); return 0; }
Output
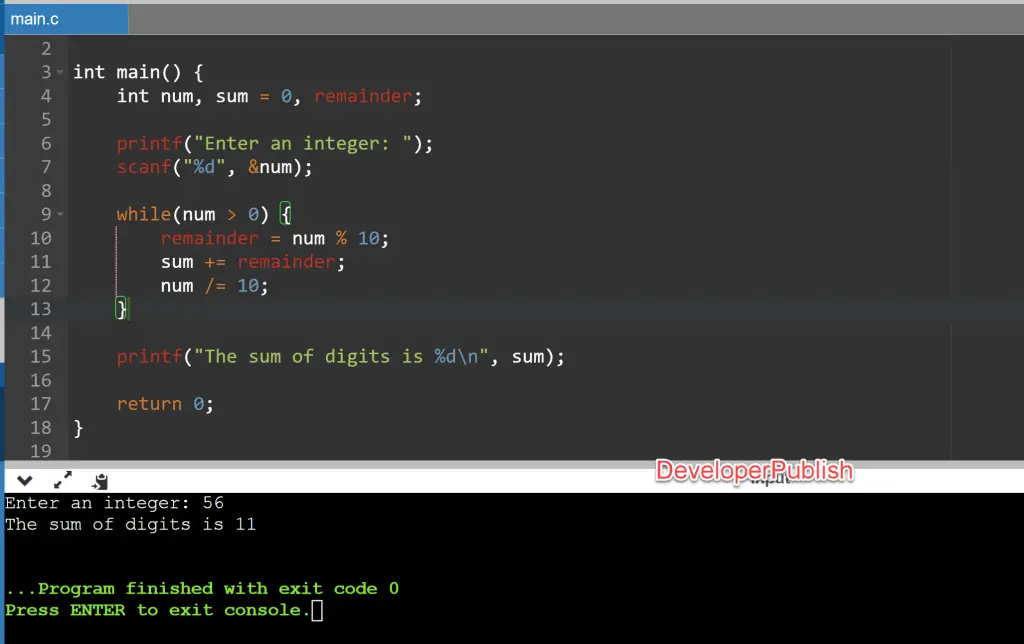
Explanation
First, we include the stdio.h
header file which is used for input and output functions. Then, we define the main()
function as the starting point of our program.
We declare three integer variables – num
, sum
, and remainder
. num
is used to store the number entered by the user, sum
is used to store the sum of the digits, and remainder
is used to store the remainder obtained after dividing num
by 10.
Next, we prompt the user to enter a number using the printf()
function. The scanf()
function is used to read in the number entered by the user. The format specifier %d
is used to specify that the input should be read as an integer.
We then use a while
loop to calculate the sum of the digits. In each iteration of the loop, we calculate the remainder of num
divided by 10 using the modulo operator %
. We add the remainder to the sum
variable, and then divide num
by 10 to remove the last digit from num
. We repeat this process until num
becomes 0.
Finally, we print the result using the printf()
function. We use the format specifier %d
to print the original number entered by the user, and %d
again to print the sum of its digits.
Conclusion
In this tutorial, we learned how to write a C program to find the sum of digits of a number. We used a while
loop to iterate through the digits of the number, and calculated the sum using the modulo operator %
and division operator /
. We also used the printf()
function to print the result to the console.