In C programming, comparing two numbers to check if they are equal is a basic operation. In this program, we will write a C program that prompts the user to enter two integers, compares them, and prints whether they are equal or not.
Problem Statement
The problem statement is to write a C program that prompts the user to enter two integers, compares them, and prints whether they are equal or not.
Solution
Here is the C program to check if two numbers are equal:
#include <stdio.h> int main() { int num1, num2; printf("Enter two integers: "); scanf("%d %d", &num1, &num2); if(num1 == num2) { printf("The two numbers are equal\n"); } else { printf("The two numbers are not equal\n"); } return 0; }
Output
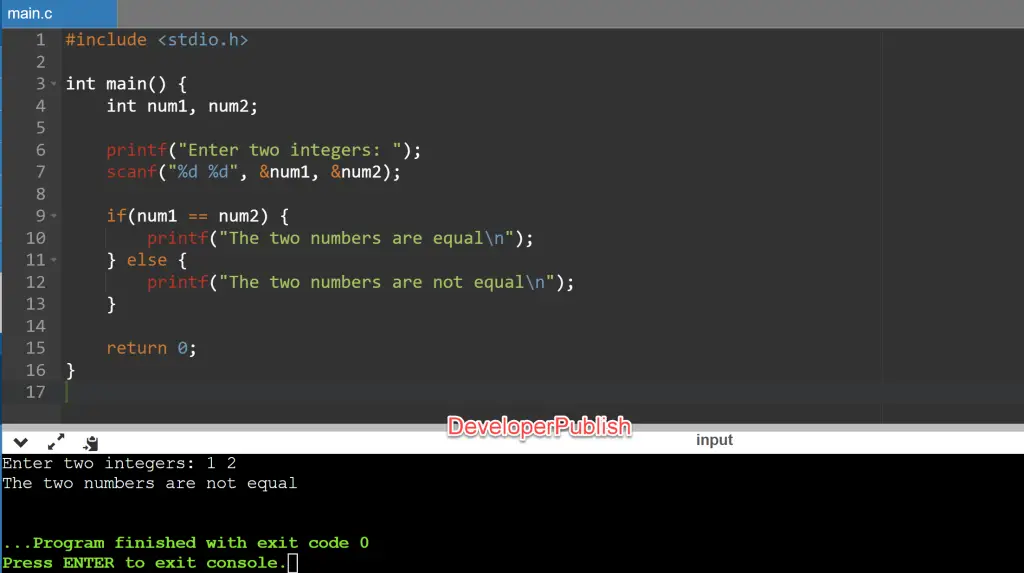
Explanation
First, we include the stdio.h
header file which is used for input and output functions. Then, we define the main()
function as the starting point of our program.
We declare two integer variables – num1
and num2
. num1
and num2
are used to store the two integers that the user inputs.
Next, we prompt the user to enter two integers using the printf()
function. The scanf()
function is used to read in the two integers entered by the user. The format specifier %d
is used to specify that the input should be read as integers.
We then use an if
statement to compare num1
and num2
. If they are equal, we print a message saying that the numbers are equal. If they are not equal, we print a message saying that the numbers are not equal.
Finally, we return 0 to indicate successful execution.