In programming, it is often necessary to find the largest number among a given set of numbers. In this program, we will write a C program that prompts the user to enter three numbers, finds the largest among them, and prints the result.
Problem Statement
The problem statement is to write a C program that prompts the user to enter three numbers, finds the largest among them, and prints the result.
Solution
Here is the C program to find the largest number among three numbers:
#include <stdio.h> int main() { int num1, num2, num3; printf("Enter the first number: "); scanf("%d", &num1); printf("Enter the second number: "); scanf("%d", &num2); printf("Enter the third number: "); scanf("%d", &num3); if (num1 >= num2 && num1 >= num3) { printf("%d is the largest number.", num1); } else if (num2 >= num1 && num2 >= num3) { printf("%d is the largest number.", num2); } else { printf("%d is the largest number.", num3); } return 0; }
Output
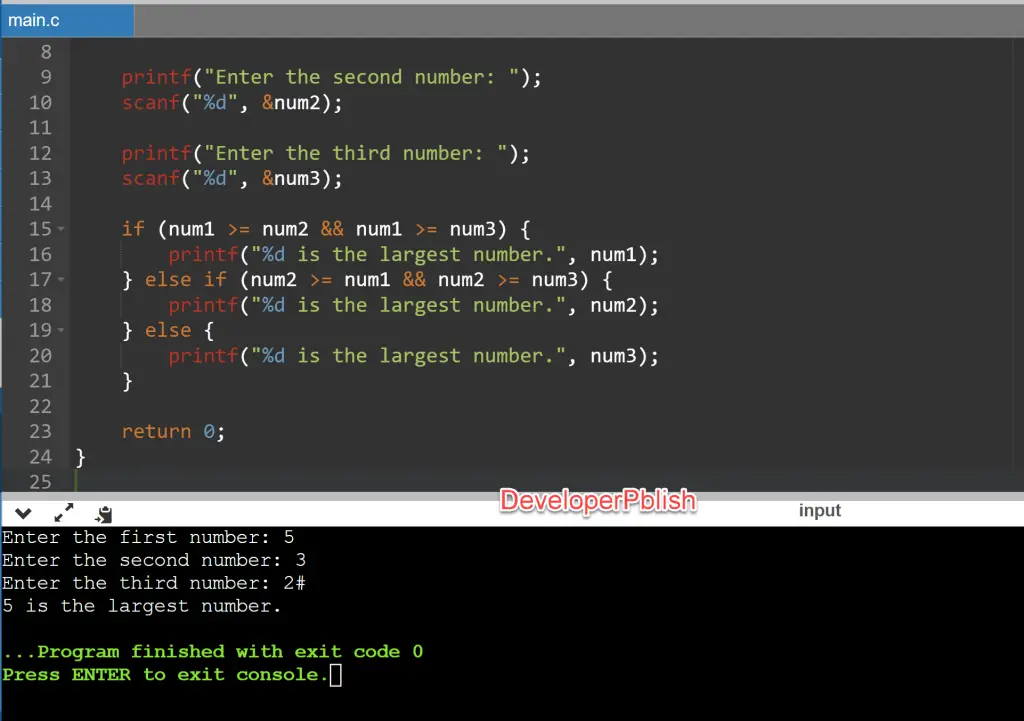
Explanation
First, we include the stdio.h
header file which is used for input and output functions. Then, we define the main()
function as the starting point of our program.
We declare three integer variables – num1
, num2
, and num3
. num1
, num2
, and num3
are used to store the three numbers entered by the user.
Next, we prompt the user to enter the first number using the printf()
function. The scanf()
function is used to read in the first number entered by the user. The format specifier %d
is used to specify that the input should be read as an integer.
We then prompt the user to enter the second number using the printf()
function. The scanf()
function is used again to read in the second number entered by the user.
We then prompt the user to enter the third number using the printf()
function. The scanf()
function is used again to read in the third number entered by the user.
We then use an if-else statement to compare the three numbers and find the largest among them. If num1
is greater than or equal to num2
and num3
, we print num1
as the largest number. If num2
is greater than or equal to num1
and num3
, we print num2
as the largest number. If neither of these conditions is true, we print num3
as the largest number.
Finally, we print the result using the printf()
function.
Conclusion
In this tutorial, we learned how to write a C program to find the largest number among three numbers. We used an if-else statement to compare the three numbers and find the largest among them. We also used the printf()
function to print the result to the console.