In programming, it is often necessary to count the number of vowels and consonants in a sentence. In this program, we will write a C program that prompts the user to enter a sentence, counts the number of vowels and consonants in the sentence, and prints the result.
Problem Statement
The problem statement is to write a C program that prompts the user to enter a sentence, counts the number of vowels and consonants in the sentence, and prints the result.
Solution
Here is the C program to count the number of vowels and consonants in a sentence:
#include <stdio.h> #include <ctype.h> int main() { char sentence[100]; int vowels = 0, consonants = 0; printf("Enter a sentence: "); fgets(sentence, 100, stdin); for (int i = 0; sentence[i] != '\0'; i++) { char ch = tolower(sentence[i]); if (ch >= 'a' && ch <= 'z') { if (ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o' || ch == 'u') { vowels++; } else { consonants++; } } } printf("Number of vowels: %d\n", vowels); printf("Number of consonants: %d\n", consonants); return 0; }
Output
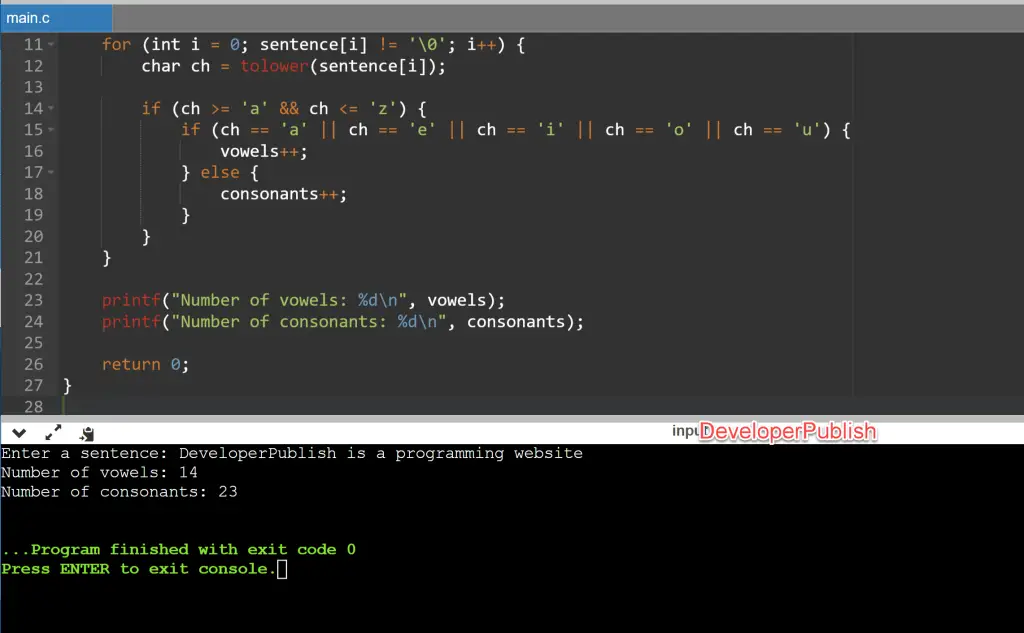
Explanation
First, we include the stdio.h
and ctype.h
header files which are used for input/output and character manipulation functions. Then, we define the main()
function as the starting point of our program.
We declare a character array sentence
with a maximum size of 100 characters to store the sentence entered by the user. We also declare two integer variables vowels
and consonants
which will be used to store the number of vowels and consonants in the sentence.
Next, we prompt the user to enter a sentence using the printf()
function. The fgets()
function is used to read in the sentence entered by the user. The first parameter to fgets()
is the character array that will store the input, the second parameter is the maximum number of characters to be read, and the third parameter is stdin
which indicates that the input should be read from the standard input (i.e., the keyboard).
We then use a for loop to iterate over each character in the sentence. We convert each character to lowercase using the tolower()
function. We then use an if-else statement to determine whether the character is a vowel or a consonant. If the character is a vowel (i.e., ‘a’, ‘e’, ‘i’, ‘o’, or ‘u’), we increment the vowels
variable. If the character is a consonant (i.e., any other character in the alphabet), we increment the consonants
variable.
Finally, we print the number of vowels and consonants using the printf()
function.
Conclusion
In this tutorial, we learned how to write a C program to count the number of vowels and consonants in a sentence. We used a character array to store the sentence entered by the user, and we used a for loop to iterate over each character in the sentence. We also used the tolower()
function to convert each character to lowercase and the isalpha()
function to check whether the character is a letter. We then used an if-else statement to determine whether the character is a vowel or a consonant, and we incremented the appropriate variable