This C# program is designed to search for a specific element within an array of integers. Searching for elements in arrays is a common task in programming, and it is often used to find the presence and location of an element in a collection of data.
Problem Statement
You are tasked with developing a program that efficiently searches for a specific element in an array of integers.
C# Program to Search an Element in an Array
using System; class Program { static void Main() { // Define an array int[] numbers = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 }; // Element to search for int target = 5; // Call the SearchElement method to check if the element exists bool found = SearchElement(numbers, target); if (found) { Console.WriteLine($"Element {target} found in the array."); } else { Console.WriteLine($"Element {target} not found in the array."); } } static bool SearchElement(int[] arr, int target) { // Iterate through the array to search for the element foreach (int element in arr) { if (element == target) { return true; // Element found } } return false; // Element not found } }
How it Works
- Initialize an Array: Create an array and populate it with elements.
- Get the Target Element: Prompt the user to enter the element they want to search for (the target element).
- Search Algorithm: Implement a search algorithm to find the target element within the array. You can choose from different search methods, such as linear search, binary search (for sorted arrays), or using built-in methods like
Array.IndexOf()
or LINQ.- Linear Search
- Using
Array.IndexOf()
- Using LINQ
- Display the Result: Based on the result of the search algorithm, display a message to the user indicating whether the target element was found and, if so, at what index.
- Repeat or Terminate: After displaying the result, you can ask the user if they want to search for another element. If yes, repeat the process; if no, terminate the program.
Input/ Output
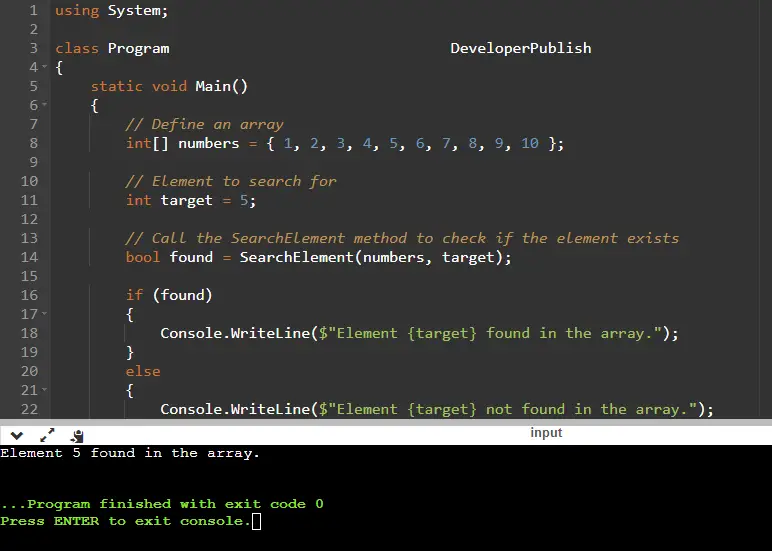