In this C# program, we will create a console application to add two matrices. Matrices are two-dimensional arrays, and adding them involves adding corresponding elements of the arrays.
Problem Statement:
Write a C# program that takes two matrices as input and calculates their sum. The program should handle matrices of different sizes, but they must have the same dimensions for addition to be valid.
C# Program to Add Two Matrices
using System; class MatrixAddition { static void Main() { Console.WriteLine("Matrix Addition Program"); Console.Write("Enter the number of rows: "); int rows = int.Parse(Console.ReadLine()); Console.Write("Enter the number of columns: "); int columns = int.Parse(Console.ReadLine()); int[,] matrixA = new int[rows, columns]; int[,] matrixB = new int[rows, columns]; int[,] sumMatrix = new int[rows, columns]; Console.WriteLine("Enter the elements of Matrix A:"); ReadMatrix(matrixA); Console.WriteLine("Enter the elements of Matrix B:"); ReadMatrix(matrixB); // Perform matrix addition for (int i = 0; i < rows; i++) { for (int j = 0; j < columns; j++) { sumMatrix[i, j] = matrixA[i, j] + matrixB[i, j]; } } Console.WriteLine("Sum of Matrix A and Matrix B:"); PrintMatrix(sumMatrix); } static void ReadMatrix(int[,] matrix) { for (int i = 0; i < matrix.GetLength(0); i++) { for (int j = 0; j < matrix.GetLength(1); j++) { Console.Write($"Enter element at position [{i + 1},{j + 1}]: "); matrix[i, j] = int.Parse(Console.ReadLine()); } } } static void PrintMatrix(int[,] matrix) { for (int i = 0; i < matrix.GetLength(0); i++) { for (int j = 0; j < matrix.GetLength(1); j++) { Console.Write(matrix[i, j] + "\t"); } Console.WriteLine(); } } }
How it Works:
- The program starts by taking the number of rows and columns for the matrices as input from the user.
- Two matrices,
matrixA
andmatrixB
, are created with the specified dimensions. - The program then reads the elements of both matrices using the
ReadMatrix
function. - It performs matrix addition by iterating through the matrices and adding corresponding elements.
- The result is stored in the
sumMatrix
. - Finally, the program prints the sum matrix using the
PrintMatrix
function.
Input/Output:
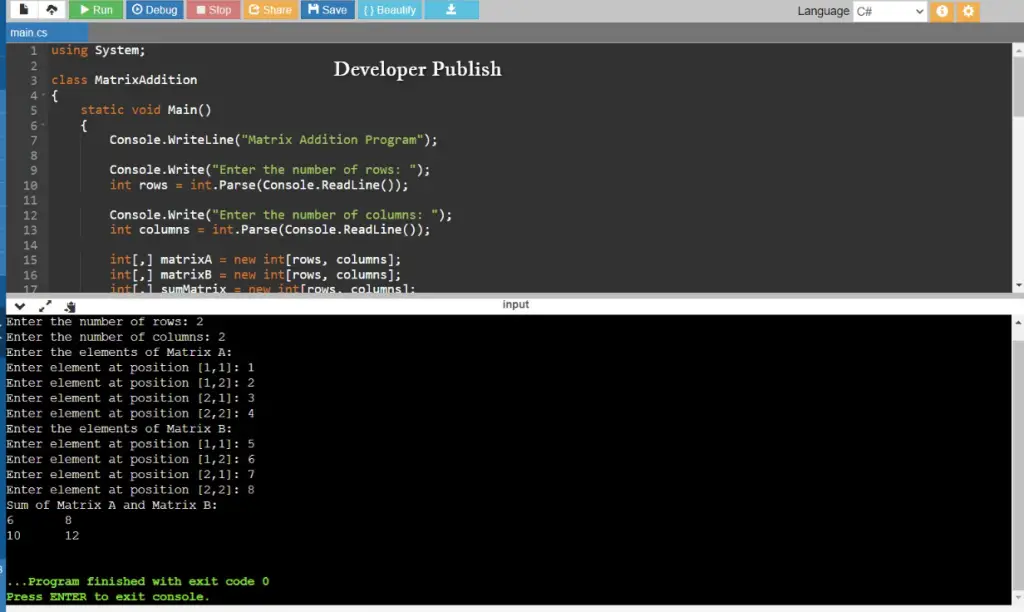