In C#, you can create a program to calculate the sum of N numbers by taking input from the user or by reading the numbers from an array. The basic idea is to use a loop to iterate through the numbers and accumulate their sum.
Problem Statement
You are tasked with creating a C# program that calculates the sum of N numbers.
C# Program to Generate the Sum of N Numbers
using System; class Program { static void Main() { Console.Write("Enter the value of N: "); if (int.TryParse(Console.ReadLine(), out int n)) { int sum = 0; for (int i = 1; i <= n; i++) { Console.Write("Enter number #{0}: ", i); if (int.TryParse(Console.ReadLine(), out int num)) { sum += num; } else { Console.WriteLine("Invalid input. Please enter a valid number."); i--; // Decrement i to re-enter the same number. } } Console.WriteLine("The sum of the {0} numbers is: {1}", n, sum); } else { Console.WriteLine("Invalid input for N. Please enter a valid integer."); } } }
How it Works
- Input N:
- The program begins by asking the user to enter an integer N, which represents the total number of values to be summed.
- Initialize Variables:
- The program initializes a variable
sum
to 0. This variable will be used to accumulate the sum of the numbers.
- The program initializes a variable
- Loop Through the Numbers:
- The program uses a
for
loop to iterate N times, starting from 1 and going up to N.
- The program uses a
- Input Numbers:
- Inside the loop, for each iteration, the program does the following:
- It either prompts the user to enter a number, or if you have an array of numbers, it accesses each element one by one.
- Inside the loop, for each iteration, the program does the following:
- Accumulate Sum:
- After obtaining a number (either from the user or the array), the program adds that number to the
sum
variable. This step accumulates the sum of the numbers as the loop iterates.
- After obtaining a number (either from the user or the array), the program adds that number to the
- Display Result:
- After the loop has completed, the program displays the final value of the
sum
variable. This value represents the sum of the N numbers entered or read from the array.
- After the loop has completed, the program displays the final value of the
- Error Handling (Optional):
- Optionally, you can include error handling to ensure that the user enters valid numbers. If an invalid input is provided (e.g., a non-numeric value), the program can display an error message and ask the user to re-enter the number.
Input/ Output
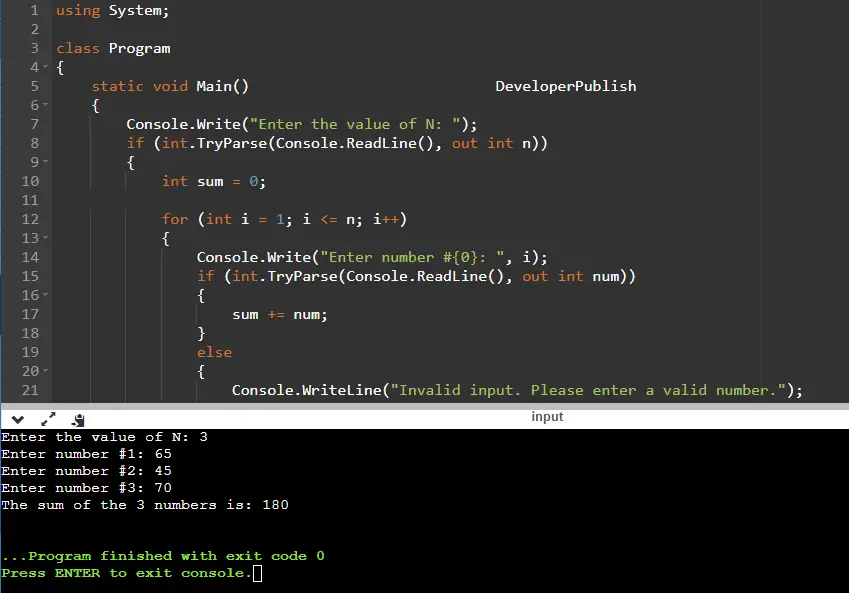