This C# program calculates the sum of the first 50 natural numbers. Natural numbers are the positive integers starting from 1.
Problem Statement
Write a C# program that calculates and displays the sum of the first 50 natural numbers. Natural numbers are positive integers starting from 1 and continuing indefinitely. Your program should use a for
loop to iterate through the first 50 natural numbers, accumulate their sum, and then output the result.
C# Program to Find the Sum of First 50 Natural Numbers using For Loop
using System; namespace SumOfFirst50NaturalNumbers { class Program { static void Main(string[] args) { int sum = 0; // Using a for loop to iterate through the first 50 natural numbers for (int i = 1; i <= 50; i++) { sum += i; } Console.WriteLine("The sum of the first 50 natural numbers is: " + sum); } } }
How it Works
- Initialization:
- We start by declaring an integer variable called
sum
and initialize it to 0. This variable will be used to store the cumulative sum of the natural numbers.
- We start by declaring an integer variable called
- For Loop:
- We use a
for
loop to perform a series of actions repeatedly. In this case, the loop will execute 50 times, starting fromi = 1
and ending wheni
becomes greater than 50. int i = 1;
initializes a loop variablei
to 1, which is the first natural number.i <= 50
is the loop condition. The loop will continue as long asi
is less than or equal to 50.i++
is the increment statement, which increasesi
by 1 after each iteration.
- We use a
- Loop Body:
- Inside the loop, we have a single statement:
sum += i;
. This statement adds the current value ofi
to thesum
variable.- In the first iteration,
i
is 1, so we add 1 tosum
. - In the second iteration,
i
is 2, so we add 2 tosum
. - This process continues until the loop completes all 50 iterations.
- In the first iteration,
- Inside the loop, we have a single statement:
- Accumulation of Sum:
- As the loop iterates, the
sum
variable accumulates the sum of the first 50 natural numbers.
- As the loop iterates, the
- Printing the Result:
- After the
for
loop finishes executing, we useConsole.WriteLine
to display the result. - We print a message, “The sum of the first 50 natural numbers is: “, followed by the value of the
sum
variable, which contains the sum we calculated in the loop.
- After the
- Program Execution:
- When you run the program, it initializes
sum
to 0, enters thefor
loop, adds the numbers from 1 to 50 tosum
, and then displays the final sum in the console.
- When you run the program, it initializes
Input/ Output
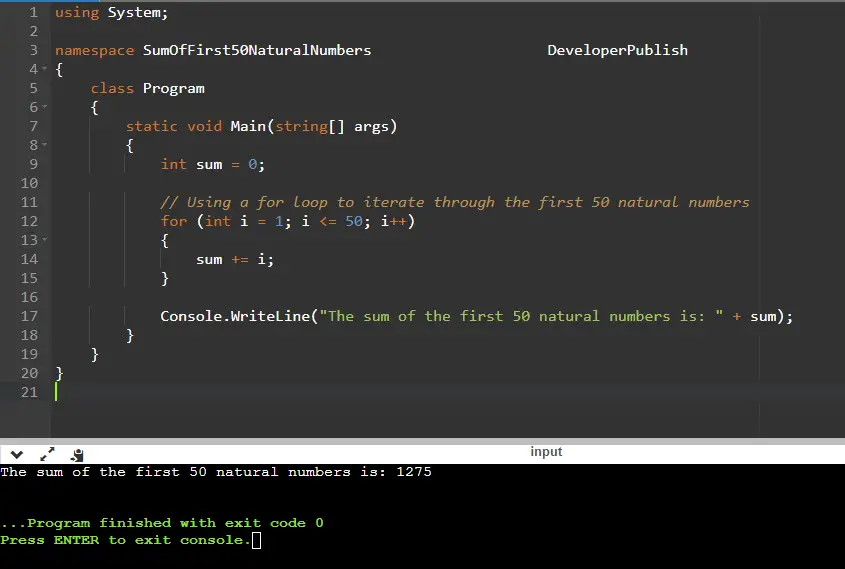