This C# program calculates the cube root of a given number using the Newton-Raphson method for approximating cube roots.
Problem statement
You need to develop a program that takes a number as input and calculates its cube root accurately.
C# Program to Find the Cube Root of a Number
using System; namespace CubeRootCalculator { class Program { static void Main(string[] args) { Console.WriteLine("Cube Root Calculator"); Console.WriteLine("===================="); Console.WriteLine("This program calculates the cube root of a number."); // Input Console.Write("Enter a number: "); double inputNumber; if (double.TryParse(Console.ReadLine(), out inputNumber)) { // Calculate cube root double cubeRoot = CalculateCubeRoot(inputNumber); // Output Console.WriteLine($"The cube root of {inputNumber} is approximately {cubeRoot:F6}"); } else { Console.WriteLine("Invalid input. Please enter a valid number."); } } // Function to calculate cube root using Newton-Raphson method static double CalculateCubeRoot(double number) { double guess = number / 3.0; // Initial guess double tolerance = 1e-6; // Tolerance for approximation while (Math.Abs(guess * guess * guess - number) > tolerance) { guess = (2.0 * guess + number / (guess * guess)) / 3.0; // Newton-Raphson formula } return guess; } } }
How it works
- The program asks the user to enter a number.
- It validates the input to ensure it is a valid numerical value.
- If the input is valid, the program calls the
CalculateCubeRoot
function to calculate the cube root. - The
CalculateCubeRoot
function uses the Newton-Raphson method to approximate the cube root. - It iteratively refines the guess until the approximation is within a specified tolerance (1e-6 in this case).
- The calculated cube root is displayed as the output.
Input / output
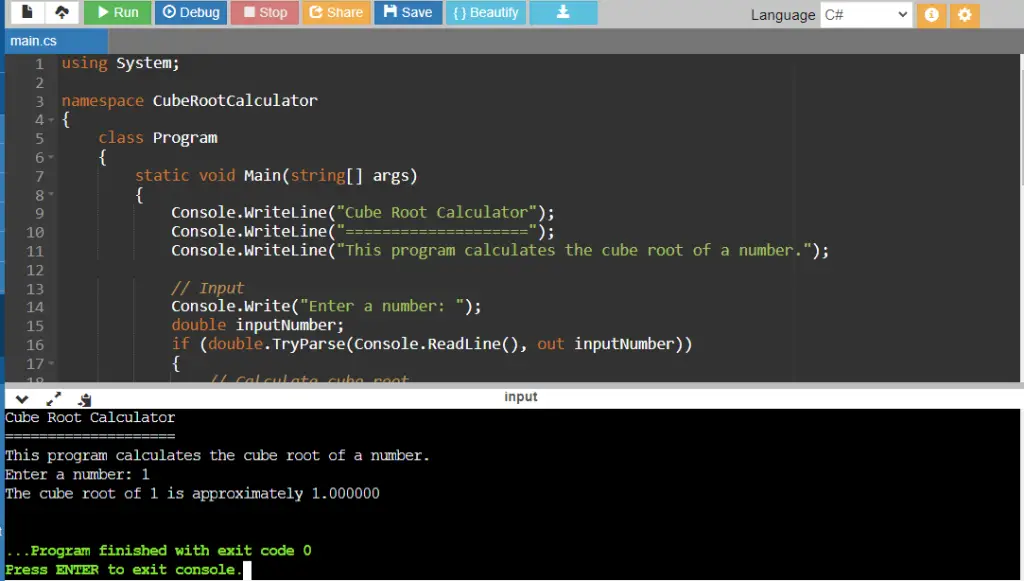