This C program converts a binary number to its decimal equivalent using the power of 2. It takes a binary number as input and returns the corresponding decimal number.
Problem statement
Write a C program to convert a binary number to its decimal equivalent.
C Program to Convert Binary to Decimal
#include <stdio.h> int binaryToDecimal(long long binaryNumber); int main() { long long binaryNumber; printf("Binary to Decimal Converter\n"); printf("Enter a binary number: "); scanf("%lld", &binaryNumber); int decimalNumber = binaryToDecimal(binaryNumber); printf("Decimal equivalent: %d\n", decimalNumber); return 0; } int binaryToDecimal(long long binaryNumber) { int decimalNumber = 0, i = 0, remainder; while (binaryNumber != 0) { remainder = binaryNumber % 10; binaryNumber /= 10; decimalNumber += remainder * (1 << i); ++i; } return decimalNumber; }
How it works?
- The program prompts the user to enter a binary number.
- The
binary To Decimal
function is called, passing the binary number as an argument. - Inside the
binary To Decimal
function, the decimal number is initialized to 0, andi
(power of 2) is initialized to 0. - The binary number is processed digit by digit in a loop until it becomes 0.
- In each iteration, the rightmost digit (
remainder
) of the binary number is extracted using the modulus operator%
. - The binary number is divided by 10 to remove the rightmost digit.
- The decimal number is updated by adding
remainder * (1 << i)
(which is equivalent toremainder * 2^i
) to it. i
is incremented by 1 to move to the next power of 2.- The process continues until the binary number becomes 0.
- Finally, the decimal number is returned.
- The decimal number is printed as the output.
Input/Output
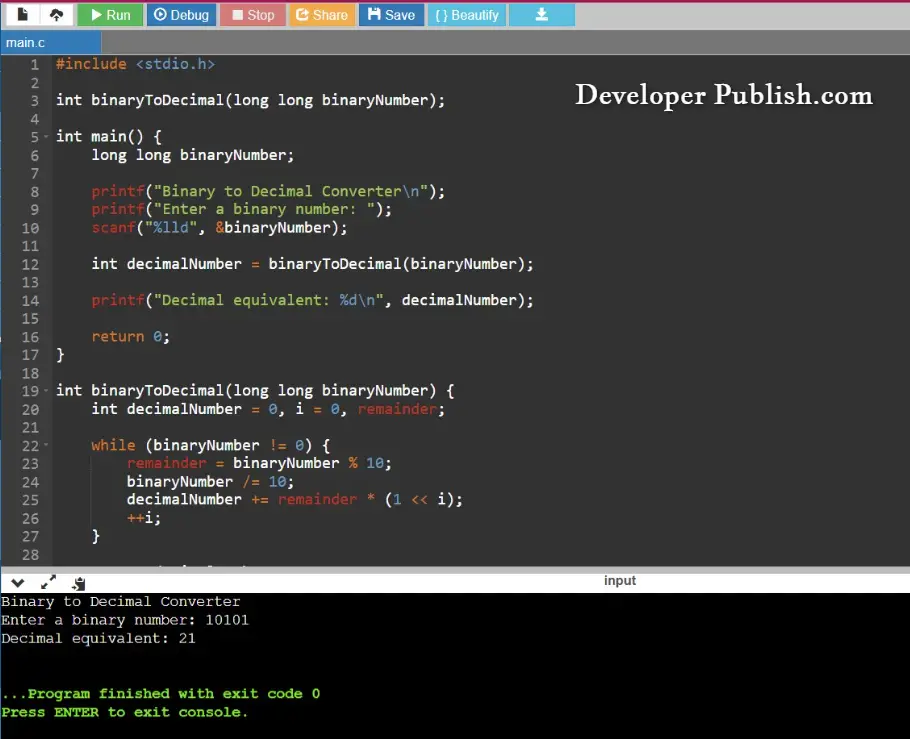