This C program prints Pascal’s triangle based on the number of rows entered by the user. Pascal’s triangle is a triangular array of numbers where each number is the sum of the two numbers directly above it.
Problem statement
Write a C program to print Pascal’s triangle based on the number of rows entered by the user.
C Program to Print Pascal Triangle
#include <stdio.h> long binomialCoeff(int n, int k) { if (k == 0 || k == n) return 1; return binomialCoeff(n - 1, k - 1) + binomialCoeff(n - 1, k); } void printPascalTriangle(int n) { for (int line = 0; line < n; line++) { for (int i = 0; i <= line; i++) printf("%ld ", binomialCoeff(line, i)); printf("\n"); } } int main() { int rows; printf("Pascal's Triangle\n"); printf("Enter the number of rows: "); scanf("%d", &rows); printf("Pascal's Triangle with %d rows:\n", rows); printPascalTriangle(rows); return 0; }
How it works?
- The program uses the
binomialCoeff
function to calculate the value of each element in Pascal’s triangle. ThebinomialCoeff
function is a recursive function that calculates the binomial coefficient using the formula C(n, k) = C(n-1, k-1) + C(n-1, k). - The
printPascalTriangle
function iterates through each line of the triangle and calls thebinomialCoeff
function to calculate the value of each element in the line. - The
main
function prompts the user to enter the number of rows they want in Pascal’s triangle. - The
printPascalTriangle
function is called with the user’s input as the argument, and it prints the Pascal’s triangle.
Input/Output
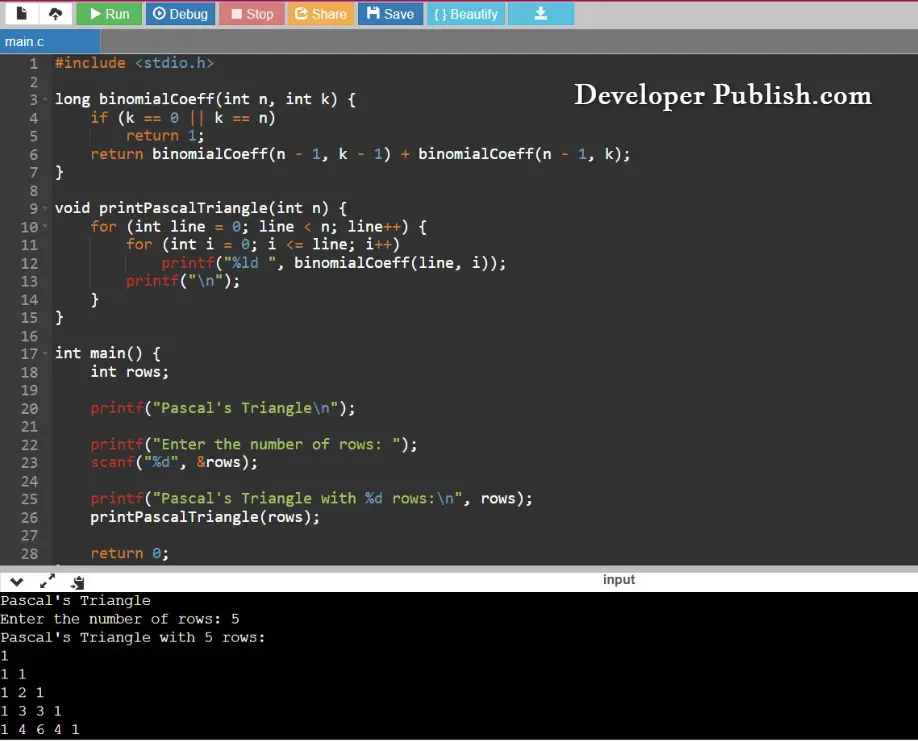