This C program checks whether a given number is a perfect number or not, where the number is entered by the user. The program then determines whether the number is a perfect number using a loop and prints a message to the screen.
Problem Statement
Write a C program that checks whether a given number is a perfect number or not, where the number is entered by the user. The program should prompt the user to enter the number, determine whether it is a perfect number using a loop, and print a message to the screen indicating whether the number is a perfect number or not.
Solution
#include <stdio.h> int main() { int n, sum = 0; // Get the value of n from user printf("Enter a positive integer: "); scanf("%d", &n); // Check whether the number is a perfect number or not for (int i = 1; i <= n / 2; i++) { if (n % i == 0) { sum += i; } } // Print the appropriate message to the screen if (sum == n) printf("%d is a perfect number.", n); else printf("%d is not a perfect number.", n); return 0; }
Output
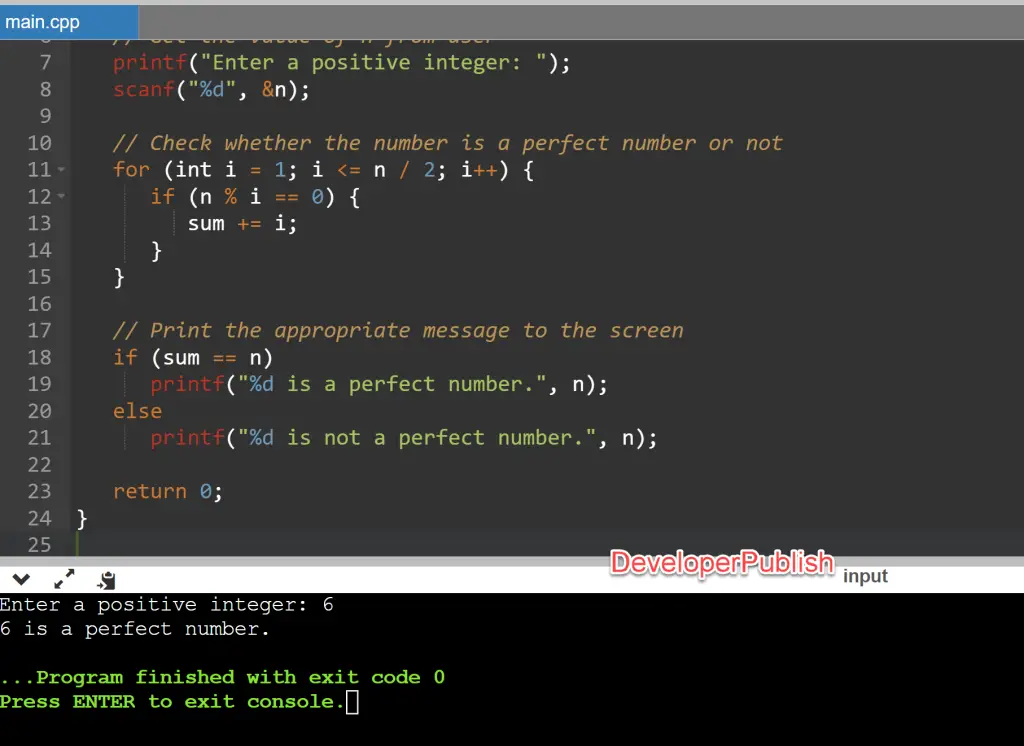
Explanation
The program starts by declaring variables to hold the value of the number entered by the user (n) and the sum of the divisors of the number (sum).
The program then prompts the user to enter a positive integer, and stores the value in the n variable.
Next, the program checks whether the number is a perfect number or not using a for loop. The loop iterates over all the positive divisors of the number from 1 to n/2. In each iteration of the loop, the program checks whether the divisor evenly divides the number using the modulo operator (%), and adds it to the sum variable if it does.
After the loop, the program checks whether the sum of the divisors is equal to the value of n. If the sum is equal to n, the program prints a message saying that the number is a perfect number. Otherwise, the program prints a message saying that the number is not a perfect number.
Finally, the program exits.
Conclusion
This C program demonstrates how to check whether a given number is a perfect number or not. It prompts the user to enter the number, determines whether it is a perfect number using a loop, and prints a message to the screen indicating whether the number is a perfect number or not.