This C program reverses a given number, where the number is entered by the user. The program then prints the reversed number to the screen.
Problem Statement
Write a C program that reverses a given number, where the number is entered by the user. The program should prompt the user to enter the number, reverse the number, and print the reversed number to the screen.
Solution
#include <stdio.h> int main() { int n, reversed_number = 0, remainder; // Get the value of n from user printf("Enter a positive integer: "); scanf("%d", &n); // Reverse the number while (n != 0) { remainder = n % 10; reversed_number = reversed_number * 10 + remainder; n /= 10; } // Print the reversed number to the screen printf("The reversed number is: %d", reversed_number); return 0; }
Output
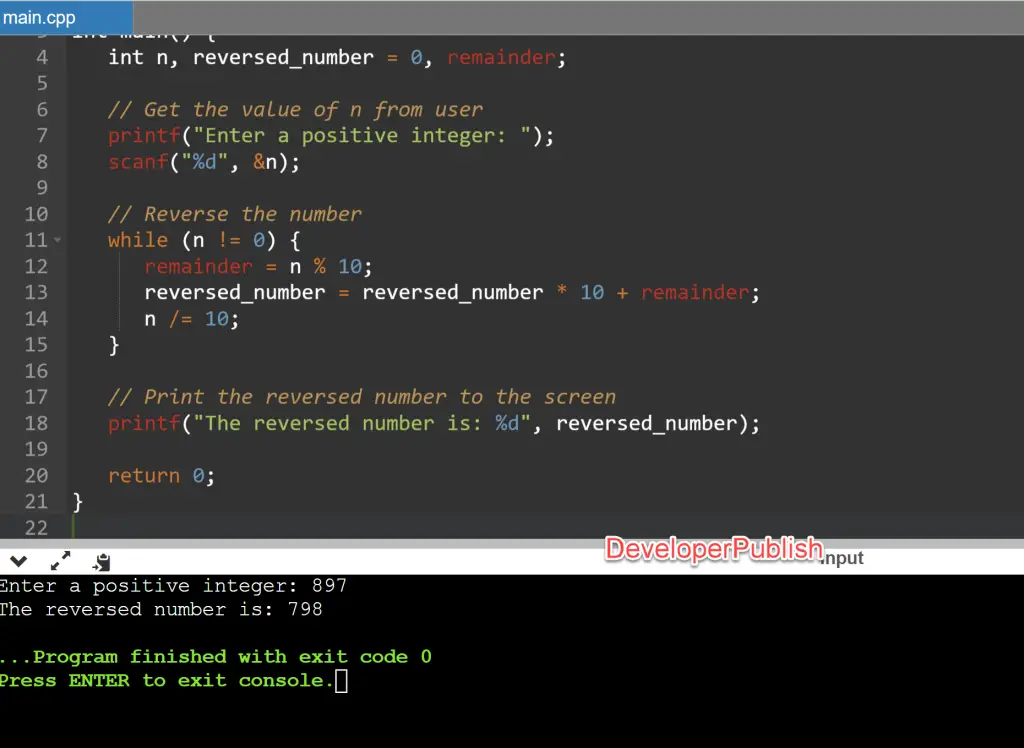
Explanation
The program starts by declaring variables to hold the value of the number entered by the user (n), the reversed number (reversed_number), and the remainder of the number when divided by 10 (remainder).
The program then prompts the user to enter a positive integer, and stores the value in the n variable.
Next, the program reverses the number using a while loop. In each iteration of the loop, the program calculates the remainder of the number when divided by 10 using the modulo operator (%), and adds it to the reversed number variable multiplied by 10 (to shift the existing digits one place to the left). The program then divides the number by 10 to remove the last digit.
After the loop, the program prints the reversed number to the screen using a printf statement.
Finally, the program exits.
Conclusion
This C program demonstrates how to reverse a given number. It prompts the user to enter the number, reverses the number, and prints the reversed number to the screen.