This C program converts an octal number to its equivalent decimal representation.
Octal is a base-8 number system that uses digits from 0 to 7. It is commonly used in computer systems and programming languages, particularly in older systems and architectures. Converting an octal number to decimal means converting it to the base-10 number system, which is the most commonly used number system. In the decimal system, digits range from 0 to 9.
Problem Statement
Write a C program that prompts the user to enter an octal number and converts it to its decimal equivalent.
The program should perform the following steps:
- Display a prompt message asking the user to enter an octal number.
- Read the octal number input from the user.
- Implement a function called
convertOctalToDecimal
that takes the octal number as an argument and returns the corresponding decimal number. - Inside the
convertOctalToDecimal
function, use the positional notation concept to convert the octal number to decimal. Multiply each digit of the octal number by powers of 8 based on its position and sum up the resulting products. - Return the decimal number from the
convertOctalToDecimal
function. - In the
main
function, call theconvertOctalToDecimal
function with the user-input octal number and store the result. - Display the decimal equivalent of the octal number on the screen.
Ensure that your program handles valid positive octal numbers properly. It should display the decimal equivalent accurately and gracefully handle any possible errors or unexpected inputs.
Note: You can assume that the user will enter valid positive octal numbers without any leading zeros.
Your program should adhere to the following specifications:
- Use appropriate variable types for storing octal and decimal numbers.
- Utilize functions to promote modularity and code reusability.
- Properly format the output to clearly display the decimal number.
C Program to Convert Octal to Decimal
#include <stdio.h> #include <math.h> int convertOctalToDecimal(int octalNumber) { int decimalNumber = 0, i = 0; while (octalNumber != 0) { decimalNumber += (octalNumber % 10) * pow(8, i); ++i; octalNumber /= 10; } return decimalNumber; } int main() { int octalNumber; printf("Enter an octal number: "); scanf("%d", &octalNumber); printf("Decimal number: %d\n", convertOctalToDecimal(octalNumber)); return 0; }
How it Works
- The program starts by displaying a prompt message asking the user to enter an octal number.
- The user enters the octal number using standard input.
- The
main
function then calls theconvertOctalToDecimal
function and passes the user-input octal number as an argument. - Inside the
convertOctalToDecimal
function, a variabledecimalNumber
is initialized to 0, which will store the decimal equivalent. - A while loop is used to extract each digit from the octal number until it becomes 0. Within the loop:
- The rightmost digit of the octal number is obtained using the modulus operator
%
with 10. - The decimal equivalent of the current digit is calculated by multiplying the digit by the corresponding power of 8. The power of 8 is determined by the position of the digit within the octal number, starting from 0 and increasing by 1 for each subsequent digit. This is achieved by using the
pow
function from the math library. - The calculated decimal value is added to the
decimalNumber
variable. - The octal number is divided by 10 to move on to the next digit.
- The rightmost digit of the octal number is obtained using the modulus operator
- Once the octal number becomes 0, the while loop exits, and the
decimalNumber
variable contains the decimal equivalent of the original octal number. - The
convertOctalToDecimal
function returns thedecimalNumber
. - Back in the
main
function, the returned decimal value is stored in a variable. - Finally, the program displays the decimal equivalent of the octal number on the screen using
printf
.
That’s how the program works! It uses the positional notation concept to convert each digit of the octal number to decimal and then sums up the results to obtain the final decimal value.
Input /Output
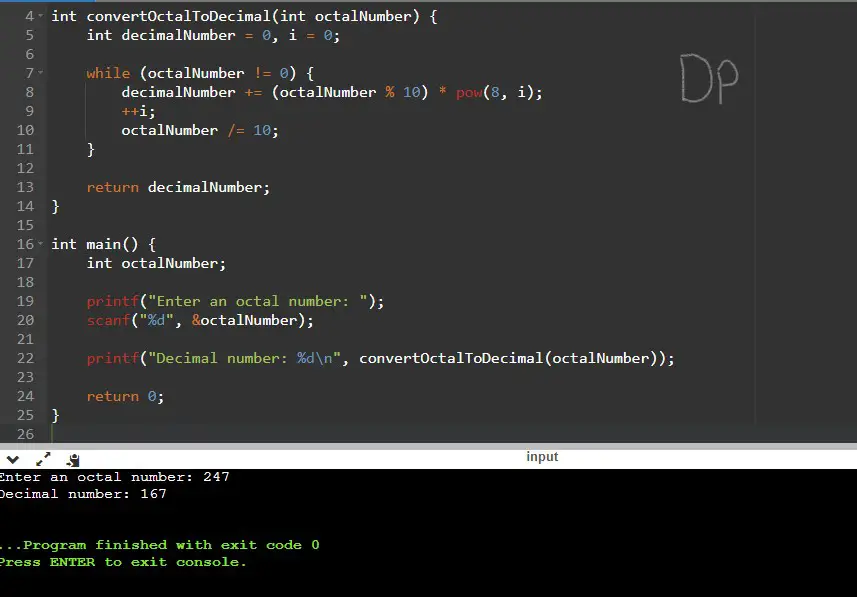
Explanation: The user is prompted to enter an octal number. In this case, the user enters “247”.
The program then converts the octal number to decimal using the convertOctalToDecimal
function.
Inside the convertOctalToDecimal
function:
- The first digit is 7, and its position is 0 (rightmost digit).
- The decimal value of the first digit (7) multiplied by 8^0 (1) is 7.
- The decimalNumber is updated to 7.
- The second digit is 4, and its position is 1.
- The decimal value of the second digit (4) multiplied by 8^1 (8) is 32.
- The decimalNumber is updated to 7 + 32 = 39.
- The third digit is 2, and its position is 2 (leftmost digit).
- The decimal value of the third digit (2) multiplied by 8^2 (64) is 128.
- The decimalNumber is updated to 39 + 128 = 167.
The convertOctalToDecimal
function returns the decimal number 167.
In the main
function, the returned decimal number is stored in a variable.
Finally, the program displays the decimal equivalent of the octal number on the screen using printf
.
So, the octal number 247 is converted to the decimal number 167.