This Python program replaces all occurrences of the character ‘a’ with ‘$’ in a given string.
Problem Statement
Write a Python program that replaces all occurrences of the letter ‘a’ with a dollar sign (‘$’) in a given string.
Your program should take a string as input and return the modified string where all occurrences of ‘a’ are replaced with ‘$’. The program should preserve the original case of the letters.
Example: Input: “apple banana avocado” Output: “$pple b$n$n$ $voc$do”
Note:
- The input string can contain uppercase and lowercase letters.
- If the input string does not contain any ‘a’ characters, the program should return the original string unchanged.
- The program should handle strings of any length.
- The program should be case-sensitive, meaning that ‘A’ should not be replaced with ‘$’.
You can implement this program using the replace()
method in Python, which allows you to replace one substring with another within a string.
Python Program to Replace All Occurrences of ‘a’ with $ in a String
def replace_characters(string): replaced_string = string.replace('a', '$') return replaced_string # Example usage input_string = 'apple banana avocado' output_string = replace_characters(input_string) print(output_string)
How it Works
The Python program works by utilizing the replace()
method to replace all occurrences of the letter ‘a’ with a dollar sign (‘$’) in the given string. Here’s how it works:
- The program defines a function called
replace_characters
that takes a string as input. - Inside the function, the
replace()
method is used on the input string to replace all occurrences of ‘a’ with ‘$’. The modified string is assigned to the variablereplaced_string
. - The modified string
replaced_string
is then returned as the output of the function. - Outside the function, an example input string is provided and assigned to the variable
input_string
. - The
replace_characters
function is called withinput_string
as the argument, and the returned value is assigned to the variableoutput_string
. - Finally, the modified string
output_string
is printed to the console.
The replace()
method is a built-in string method in Python that takes two arguments: the substring to be replaced and the substring to replace it with. In this case, ‘a’ is specified as the substring to be replaced, and ‘$’ is specified as the substring to replace it with.
The replace()
method scans the input string and replaces all occurrences of ‘a’ with ‘$’, creating a new modified string. The original string remains unchanged. The function replace_characters
encapsulates this logic and allows for reusability.
Input/ Output
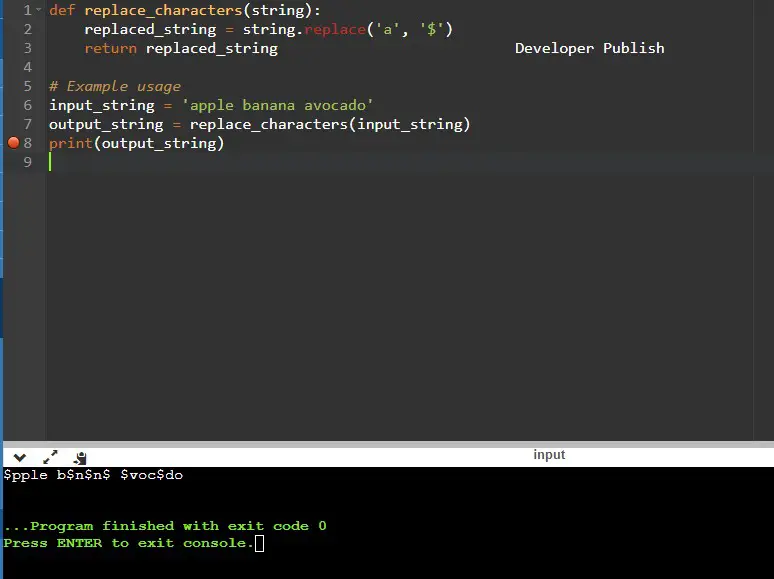