This Python program replaces every blank space in a given string with a hyphen ‘-‘.
Program Statement
Write a Python program that replaces every blank space with a hyphen (“-“) in a given string.
The program should:
- Accept a string as input from the user.
- Replace every occurrence of a blank space (” “) with a hyphen (“-“) in the input string.
- Print the modified string as the output.
The program should be able to handle strings of any length and can be used for various applications where replacing blank spaces with hyphens is required.
You can implement this program using the replace()
method in Python, as demonstrated in the earlier code example.
Python Program to Replace Every Blank Space with Hyphen in a String
def replace_spaces_with_hyphen(string): replaced_string = string.replace(" ", "-") return replaced_string # Example usage input_string = "Hello World! This is a test." output_string = replace_spaces_with_hyphen(input_string) print(output_string)
How it works
Let’s go through how the Python program works:
- The program starts by defining a function called
replace_spaces_with_hyphen
that takes a string as input. - Inside the function, the
replace()
method is used on the input string. Thereplace()
method takes two arguments: the substring to be replaced and the substring to replace it with. In this case, we want to replace every occurrence of a blank space (” “) with a hyphen (“-“), so we pass these two values as arguments to thereplace()
method. - The modified string is then assigned to the variable
replaced_string
. - Finally, the
replaced_string
is returned as the output of the function. - After defining the function, the program prompts the user to enter a string by using the
input()
function. The entered string is stored in the variableinput_string
. - The program then calls the
replace_spaces_with_hyphen()
function, passing theinput_string
as an argument. The function is executed, and the modified string is returned. - The modified string is assigned to the variable
output_string
. - Lastly, the program prints the
output_string
using theprint()
function, displaying the string with hyphens replacing the blank spaces.
By following these steps, the program takes a string as input, replaces the blank spaces with hyphens using the replace()
method, and then prints the modified string as the output.
Input/Output
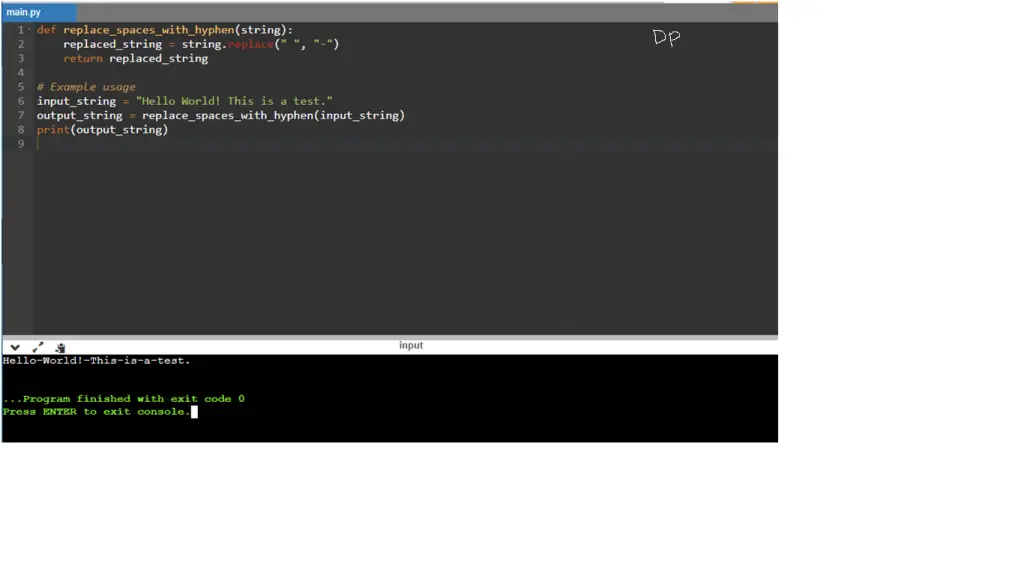