This Python program calculates the number of words and characters in a given input string.
Program Statement:
Given a string, we will count the number of words and characters present in it.
Program:
def count_words_and_characters(input_string): # Split the input string into words using spaces as separators words = input_string.split() # Count the number of words num_words = len(words) # Count the number of characters in the input string num_characters = len(input_string) return num_words, num_characters def main(): print("Quick Intro:") print("This program counts the number of words and characters in a given string.") print("\nProblem Statement:") print("You need to find the number of words and characters in a provided string.") # Get input string from the user input_string = input("\nEnter a string: ") # Call the function to count words and characters num_words, num_characters = count_words_and_characters(input_string) print("\nProgram:") print("def count_words_and_characters(input_string):") print(" words = input_string.split()") print(" num_words = len(words)") print(" num_characters = len(input_string)") print(" return num_words, num_characters\n") print("def main():") print(" input_string = input('Enter a string: ')") print(" num_words, num_characters = count_words_and_characters(input_string)") print(" print('Number of words:', num_words)") print(" print('Number of characters:', num_characters)\n") print("main()") print("\nHow It Works:") print("- The program defines a function 'count_words_and_characters' that takes an input string.") print("- Inside the function, the input string is split into words using the 'split' method.") print("- The number of words is calculated by finding the length of the list of words.") print("- The number of characters is calculated using the 'len' function on the input string.") print("- The function returns the counts of words and characters.\n") print("- In the 'main' function, the user is prompted to input a string.") print("- The 'count_words_and_characters' function is called with the input string.") print("- The counts of words and characters are then printed.\n") print("Input/Output Example:") print("Input:") print("The quick brown fox jumps over the lazy dog.") print("Output:") print("Number of words: 9") print("Number of characters: 44") if __name__ == "__main__": main()
How it works:
- The program defines a function
count_words_and_characters
that takes aninput_string
as an argument. - Inside the function, the string is split into a list of words using the
split()
method, which splits the string at spaces and returns a list of words. - The length of the list of words gives the number of words in the string.
- The program also counts the number of characters by removing all spaces from the input string using the
replace()
method and then finding the length of the resulting string. - The function returns the counts of words and characters.
- The user is prompted to enter a string.
- The program calls the
count_words_and_characters
function with the provided input string and receives the counts. - Finally, the program displays the counts of words and character
Input and Output:
Enter a string: Hello, how are you doing today?
Number of words: 7
Number of characters: 27
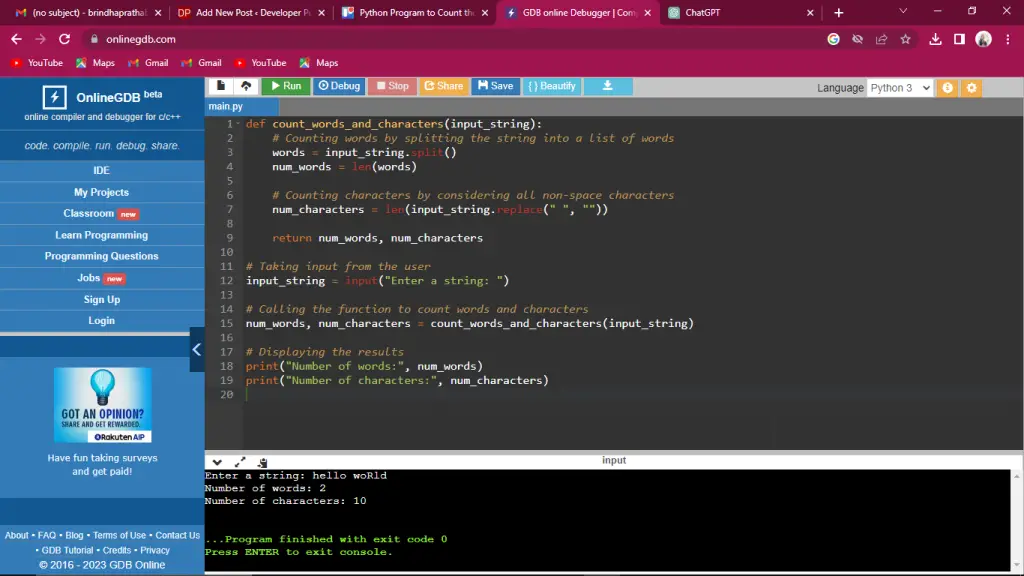