Lists are versatile data structures in Python that allow you to store and manipulate collections of items. This program demonstrates how to create a Python program that removes the ith occurrence of a given word from a list.
Problem Statement
Write a Python program that takes a list of words, a target word, and an index i as input. The program should remove the ith occurrence of the target word from the list.
Python Program to Remove the ith Occurrence of a Given Word in a List
def remove_ith_occurrence(word_list, target_word, index): occurrences = 0 new_list = [] for word in word_list: if word == target_word: occurrences += 1 if occurrences == index: continue new_list.append(word) return new_list # Input word_list = input("Enter a list of words (comma-separated): ").split(',') target_word = input("Enter the target word to remove: ") occurrence_index = int(input("Enter the occurrence index to remove: ")) # Removing the ith occurrence of the target word new_word_list = remove_ith_occurrence(word_list, target_word, occurrence_index) # Output print("Modified word list:", new_word_list)
Input / Output
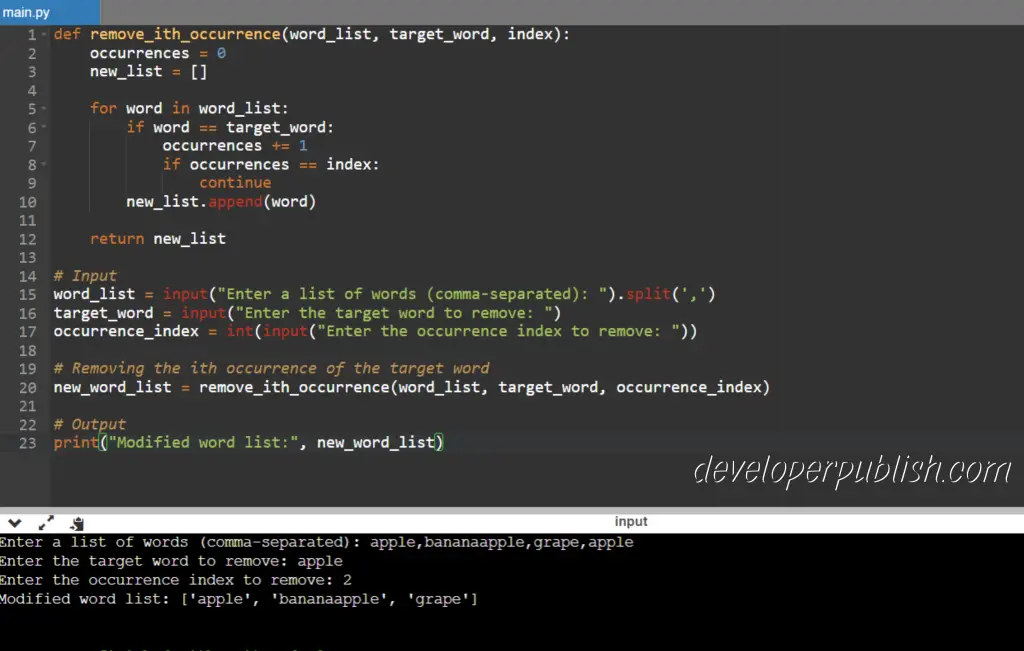