In finance, a binomial tree is a graphical representation used to model the price movement of financial instruments over time. It’s particularly useful for pricing options and other derivatives. This program demonstrates the implementation of a simple binomial tree in Python.
Problem Statement
Implement a binomial tree to calculate the option price using the European option pricing model. The program should take the necessary parameters such as the current stock price, strike price, time to maturity, risk-free interest rate, volatility, and the number of time steps in the tree.
Python Program to Implement Binomial Tree
import math def binomial_tree_option_pricing(S, K, T, r, sigma, n): dt = T / n u = math.exp(sigma * math.sqrt(dt)) d = 1 / u p = (math.exp(r * dt) - d) / (u - d) option_values = [[0 for _ in range(n+1)] for _ in range(n+1)] for j in range(n+1): option_values[n][j] = max(0, S * (u ** (n - j)) * (d ** j) - K) for i in range(n-1, -1, -1): for j in range(i+1): option_values[i][j] = math.exp(-r * dt) * (p * option_values[i+1][j] + (1-p) * option_values[i+1][j+1]) return option_values[0][0] # Input S = float(input("Enter current stock price: ")) K = float(input("Enter strike price: ")) T = float(input("Enter time to maturity (in years): ")) r = float(input("Enter risk-free interest rate: ")) sigma = float(input("Enter volatility: ")) n = int(input("Enter number of time steps: ")) option_price = binomial_tree_option_pricing(S, K, T, r, sigma, n) print(f"Option price: {option_price:.2f}")
How It Works
- The program defines a function
binomial_tree_option_pricing
which calculates the option price using the binomial tree model. - The function takes parameters such as the current stock price (
S
), strike price (K
), time to maturity (T
), risk-free interest rate (r
), volatility (sigma
), and the number of time steps (n
). - The function calculates the upward and downward movement factors (
u
andd
) and the risk-neutral probability (p
). - It initializes a 2D list
option_values
to store option values at each node in the tree. - The option values at maturity are calculated and stored in the last row of the
option_values
matrix. - Backward induction is used to calculate the option values at earlier time steps.
- The calculated option price is printed as output.
Input/Output
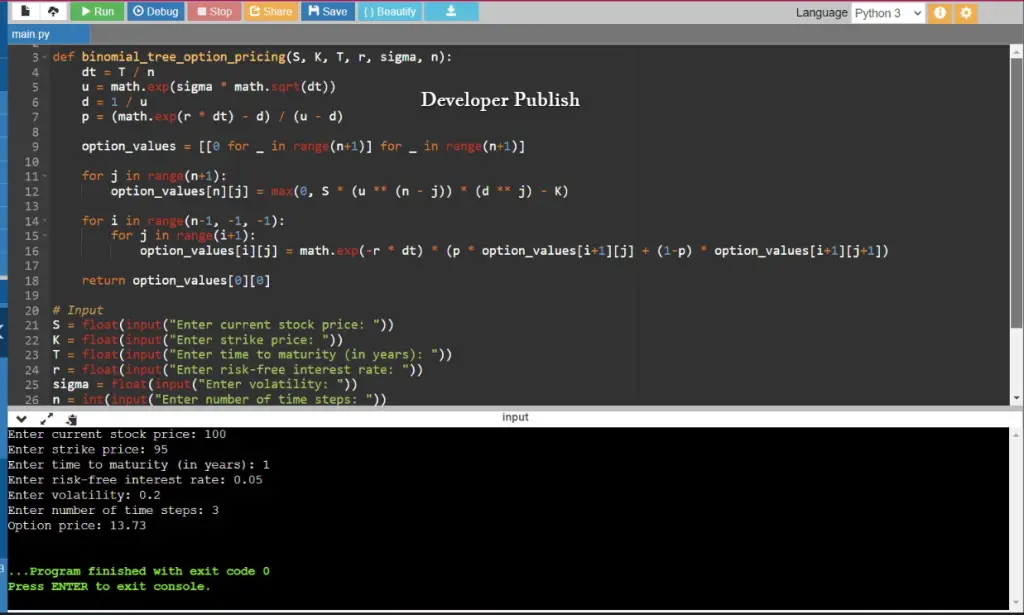